Overview
MODdy is a desktop application used by NUS Computing students to track their course progression, manage modules and manage deadlines. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 10 kLoC. It is an application developed by a team of 5 NUS Computing students, adapted from AddressBook-Level3 project created by SE-EDU initiative at SE-Education.
Summary of contributions
-
Major enhancement: Added the ability to show profile, module, semester and focus area
-
What it does: Allows the user to display an overview of his profile, display details of a module, display all modules taken in the specified semester, and display all modules available under the specified focus area.
-
Justification: This feature improves the product significantly because it allows a user to see all his modules and grades at one glance, and this convenience is a main feature of this app. It also allows user to see the modules he has taken, information on each module and requirements of each focus area so they can plan for modules to take better.
-
Highlights: This enhancement required an in-depth analysis of design alternatives as there were many different objects (e.g.
Name
,ModuleCode
,Year
,FocusArea
) to be parsed separately. The implementation too was challenging as it was decided to have a singleShowCommand
class instead of extracting eachshow
feature to inherit aShowCommand
class.
-
-
Major enhancement: Added the ability to store modules under each course requirements, as well as modules under each focus areas in JSON format
-
What it does: Allows the app to parse the course data into relevant Java objects, and to be used for the implementations of the app’s main features.
-
Justification: This feature improves the product significantly because it allows the app to provide users with all of SoC courses' information from different websites in a single app, which is a main feature of this app.
-
Highlights: This enhancement required the process of going through each of the course’s website under NUS School of Computing, as well as manually extracting and converting information provided by NUS SoC into the JSON file.
-
-
Minor enhancement: Added the ability to manage tasks with deadlines by implementing deadlines related classes.
-
Code contributed: Functional and test code
-
Other contributions:
-
Project management:
-
Managed all 5 milestones
v1.0
-v1.4
on GitHub systematically, linking relevant issues and pull requests for each of them (Milestone v1.0, v1.1, v1.2, v1.3, v1.4). -
Maintained the entire issue tracker by opening issues for each milestone, tagging with the appropriate labels, assigning issues to members and milestones, authoring up to 79 issues.
-
-
Team-based tasks:
-
Wrote User Guide sections that are not specific to features implemented by each member, e.g. Introduction, About the user guide, Quick Start, FAQ, Command Summary.
-
Wrote Developer Guide sections that are not specific to features implemented by each member, e.g. Introduction, Setting up, Design: Architecture.
-
Did major cosmetic tweaks to revamp the User Guide, redesigning the way information is presented in the User Guide (Pull requests #150, #156, #160).
-
-
Enhancements to existing features:
-
Community:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide for features implemented. They showcase my ability to write documentation targeting end-users. |
Creating new user profile : new
If you want to get started on using MODdy, you will have to create a new profile. By using this command, it creates a profile for you as shown in the figure below. You will have to provide your details as parameters.
Format: new n/name c/course y/year.semester [f/focusArea]
Example: new n/John c/Computer Science y/2.2
creates a new profile with the name "John", currently majoring in "Computer Science" and is a Year 2 Semester 2 student, as shown in Figure 4 below.
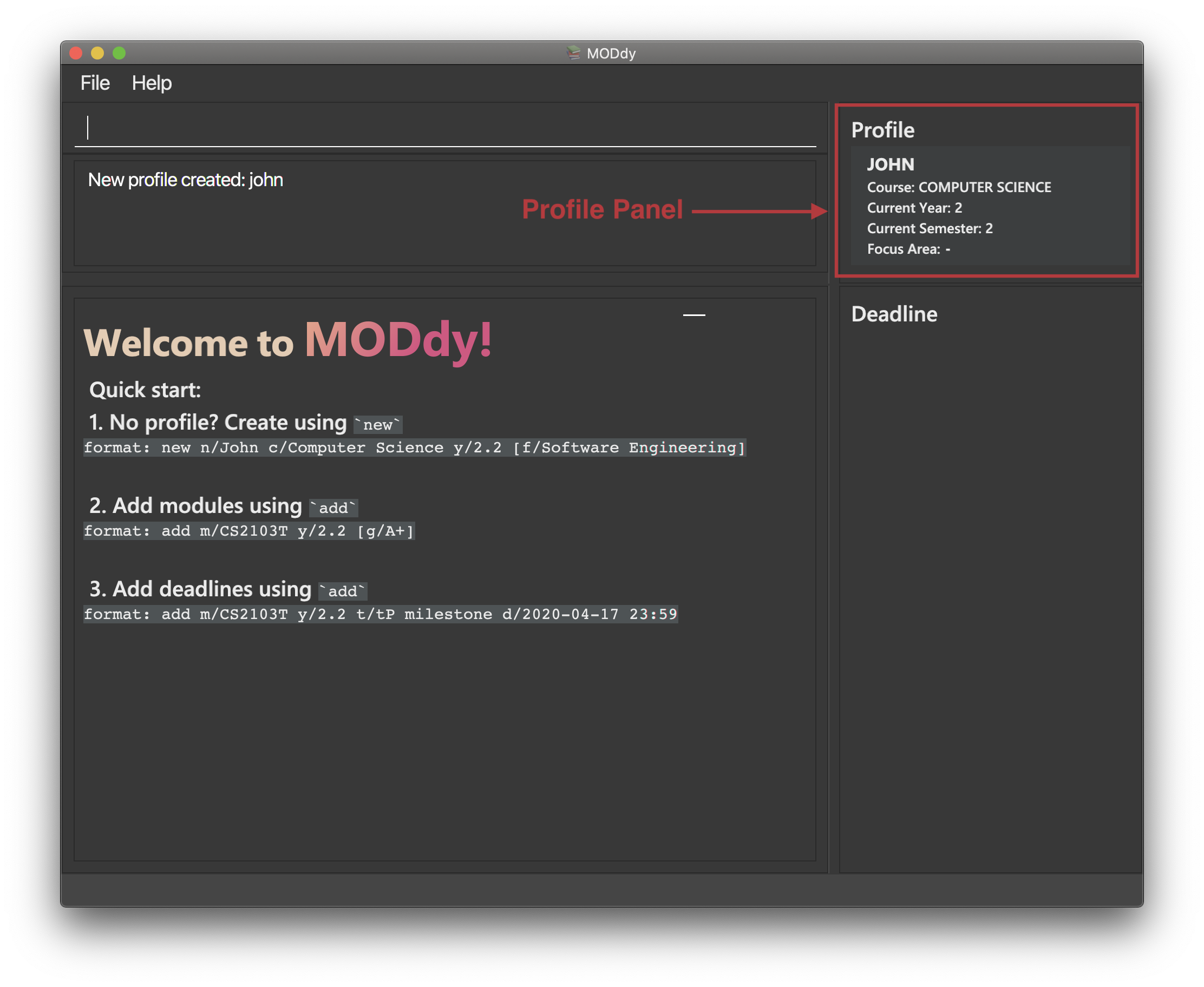
new n/John c/Computer Science y/2.2 f/Software Engineering If you know your focus area, using this command will add focus area "Software Engineering" to your new profile alongside other details. |
The maximum Year and Semester you can go up to is Year 8 Semester 2. |
Adding module or task: add
If you want to add modules to each of your semesters or add tasks to each of your modules in the current semester, this command is the right one for you!
There are two ways you can use the add
command:
Adding a module to MODdy
Format: add m/moduleCode y/year.semester [g/grade]
You can add multiple modules at the same time but only to the same year and semester. To add multiple modules, just append the m/moduleCode tags right after another, e.g. add m/CS1231 m/IS1103 m/MA1521 y/1.1 .
|
However, you cannot add grades when adding multiple modules. |
Example: add m/CS2103T y/2.2
adds CS2103T to Year 2 Semester 2, as shown in Figure 5 below.
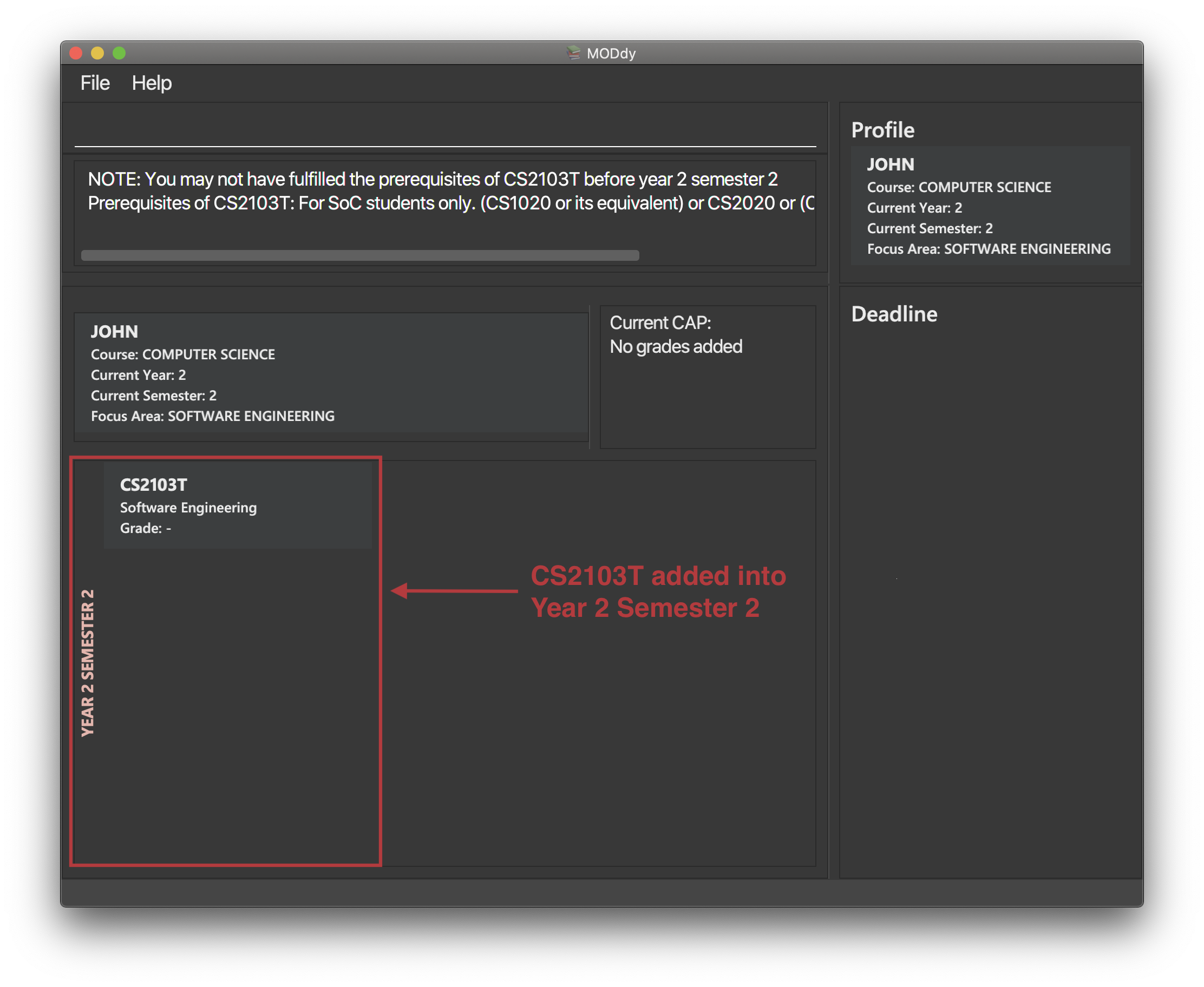
add m/CS2105 y/2.1 g/A+ You can add CS2105 with its resulting grade, A+, concurrently to the list of modules under Year 2 Semester 1. |
You can add up to a maximum of 10 modules per semester in MODdy. |

Adding a task with a deadline to a module in MODdy
Format: add m/moduleCode t/task [d/deadline]
You can add multiple tasks at once but only to the same module, e.g. add m/CS1231 t/tutorial d/2020-04-20 18:00 t/assignment d/2020-04-25 23:59 . |
However, for multiple tasks, as long as one task has a deadline, all t/task tags have to be appended with d/deadline tags.For the tasks with no deadlines, the tag can just be d/ , e.g. add m/IS1103 t/project d/2020-05-01 23:59 t/reflection d/ .
|
Example: add m/CS2105 t/Assignment d/2020-04-20 23:59
adds a task named "Assignment"
with the deadline "20 April 2020 23:59" to the already-existing module CS2105, as shown in Figure 7 below.
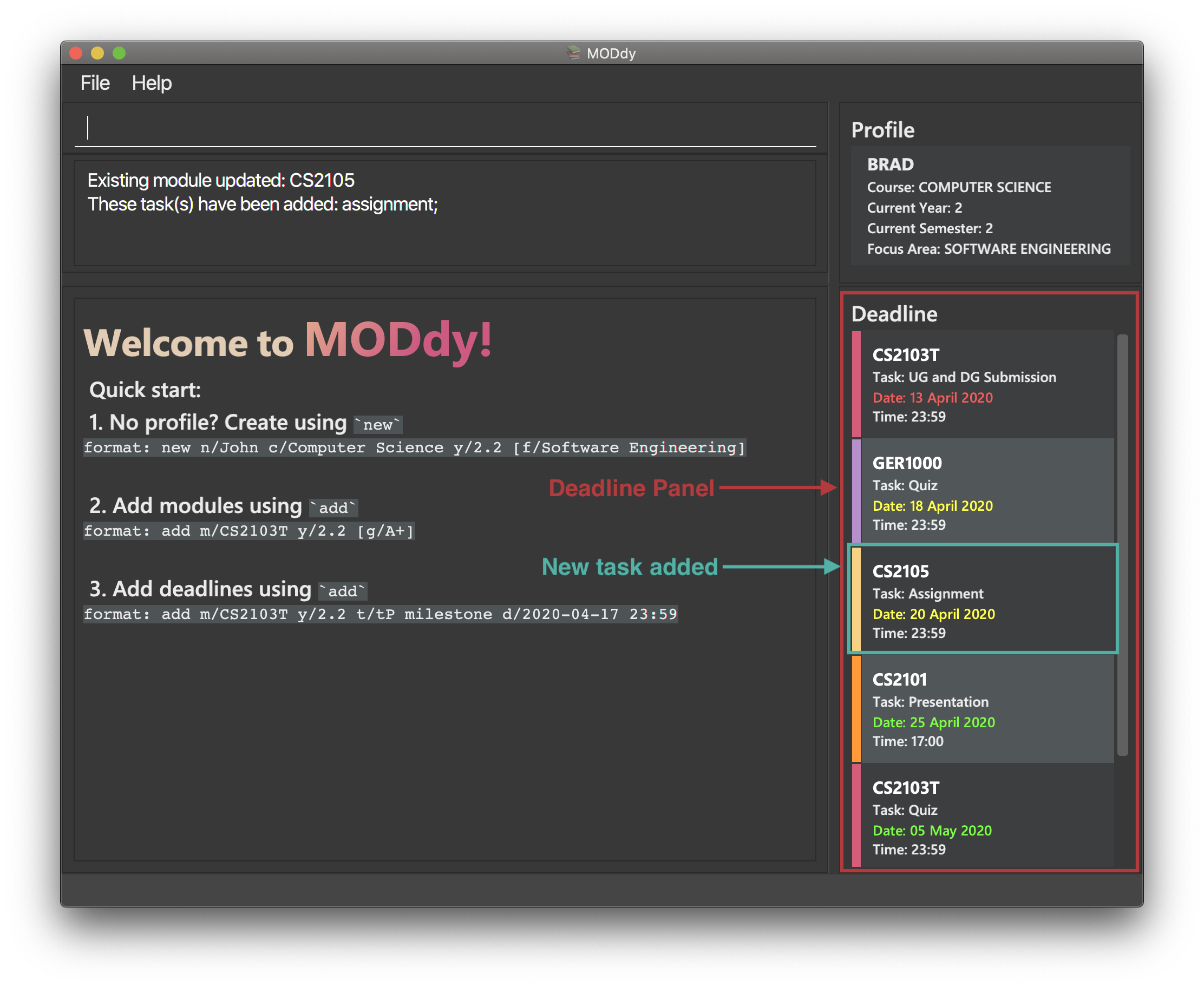
Dates are highlighted and sorted according to the number of days remaining as shown in Figure 7 above. Red: 0 - 5 days Orange: 6 - 10 days Green: ≥ 11 days |
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide for features implemented. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Structure of Command Features
The Command
features in MODdy all extends from a single abstract class Command
. A specific Command
object created is executed by the LogicManger
using the respective Parser
, which then returns a CommandResult
object and provides the result of that Command
feature.
The following class diagram shows the implementation of MODdy’s Command
features:
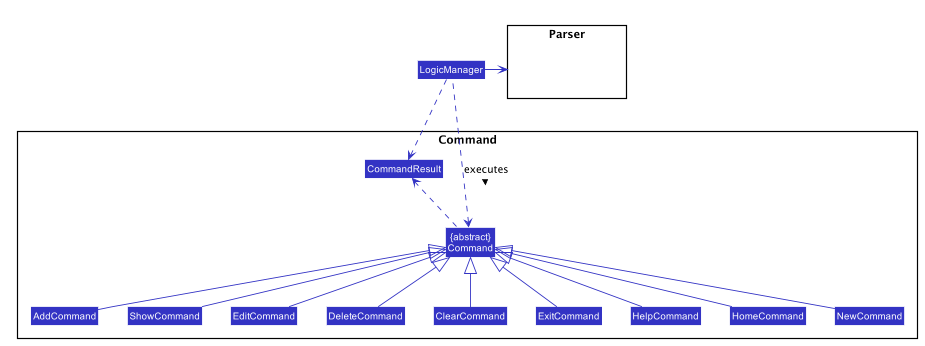
Command
The sections below gives more details on each Command
feature.
New Profile Feature
The new
feature allows the user to create a Profile
with the command new
, appended with the tags.
The tags are:
-
n/name
forName
-
c/course
forCourse
-
y/currentYear.currentSemester
for current year and semester -
f/focusArea
forFocusArea
Current Implementations
NewCommand
extends from the Command
class and uses the inheritance to facilitate the implementation. NewCommand
is parsed using NewCommandParser
to split the user input into relevant fields.
The following sequence diagram shows how the new
operation works with the input: new n/John c/Computer Science y/2.2
.
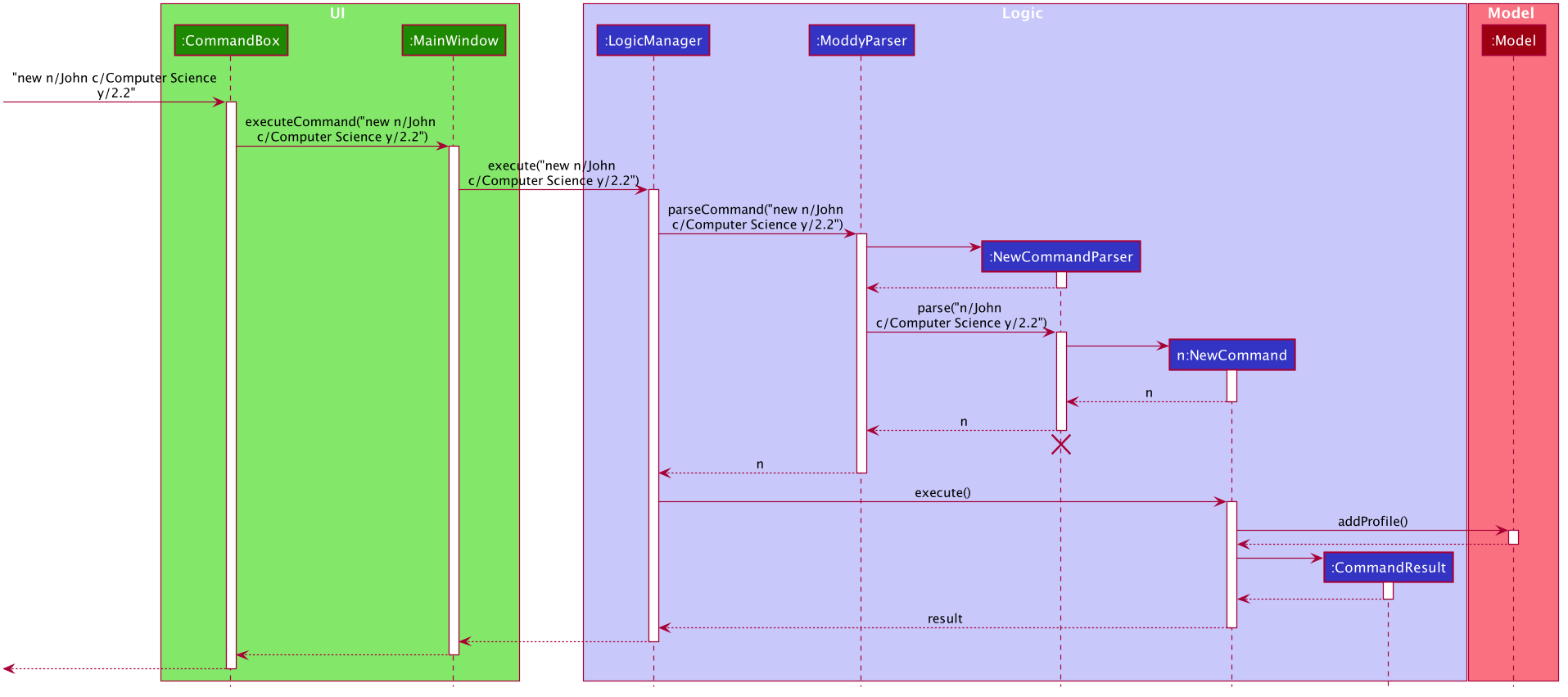
new n/John c/Computer Science y/2.2
commandDesign Considerations
-
Alternative 1 (current choice): Use the
y/year.semester
tag like all other commands-
Pros: More intuitive for user (only one tag related to year and semester).
-
Cons: Harder to convey the idea that it has to be current year and current semester to user.
-
-
Alternative 2: Have an additional tag
cs/currentYear.currentSemester
instead ofy/year.semester
to identify the year and semester user is in-
Pros: Easier to differentiate the current year and semester user is in among all
y/year.semester
tags. -
Cons: More tags to parse, and also less intuitive for user (have to use both
cs/
andy/
).
-
Eventually, we decided on alternative 1 to prioritize user-friendliness and cleaner code.
Add Module / Task Feature
The add
feature allows the user to add a Module
and a Task
with a Deadline
for an existing module with the command add
, appended with the tags.
The tags are:
-
m/moduleCode y/year.semester [g/grade]
for adding a module -
m/moduleCode y/year.semester t/task [d/deadline]
for adding a task to an existing module
Multiple modules can be added at once but only to the same year and semester, e.g. add m/CS1231 m/IS1103 m/MA1521 y/1.1 .Multiple tasks can be added at once but only to the same module, e.g. add m/CS1231 t/tutorial d/2020-04-20 18:00 t/assignment d/2020-04-25 23:59 . |
g/grade is optional.However, grades cannot be added when adding multiple modules. |
d/deadline is optional.However, for multiple tasks, as long as one Task has a Deadline , all t/task tags have to be appended with d/deadline tags. For the tasks with no deadlines, the tag can just be d/ .
|
Current Implementations
AddCommand
extends from the Command
class and uses the inheritance to facilitate the implementation. AddCommand
is parsed using AddCommandParser
to split the user input into relevant fields.
The following sequence diagram shows how the add
operation works with input: add m/CS2105 y/2.2
.
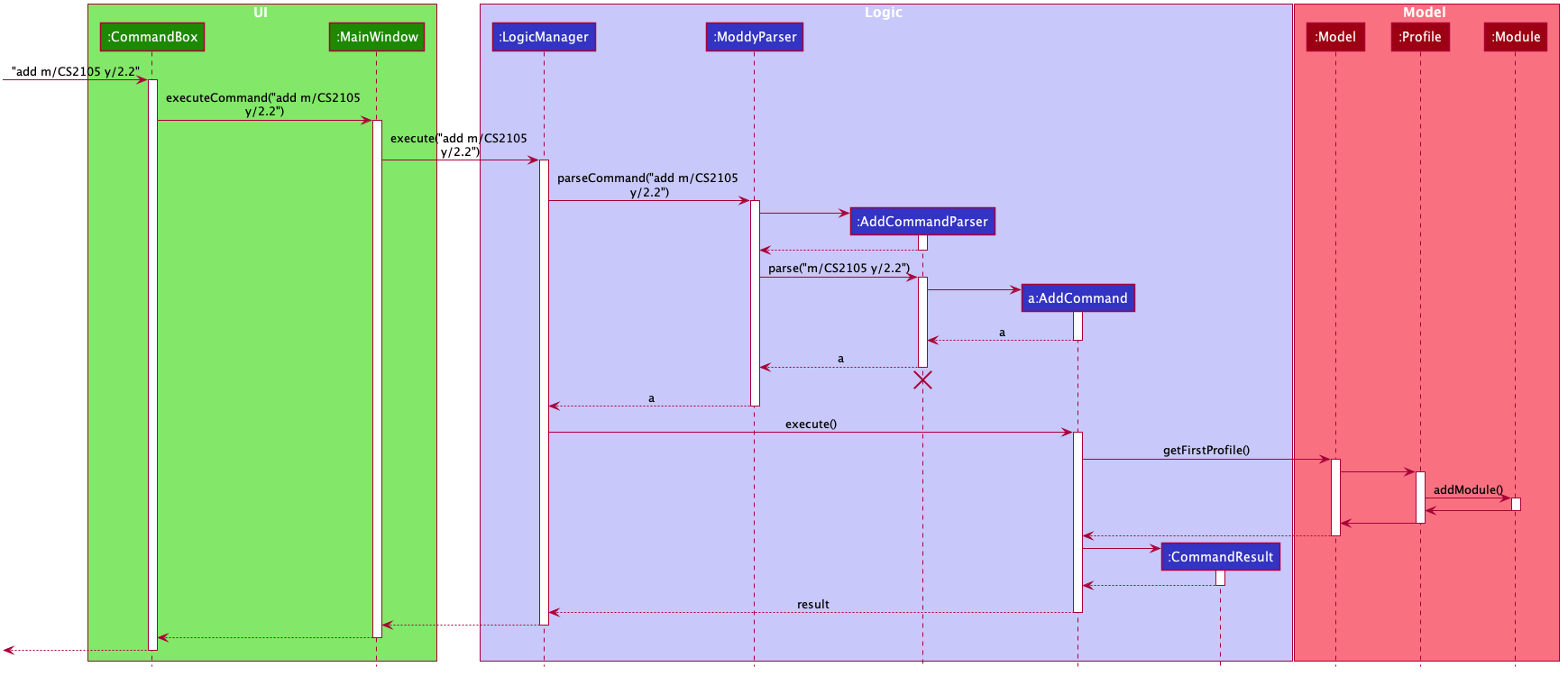
add m/CS2105 y/2.2
commandDesign Considerations
Aspect 1: How modules are added
-
Alternative 1 (current choice): Add multiple
Module
objects with oneAddCommand
-
Pros: More convenient for the user.
-
Cons: Inconsistent with other commands, which can only take in one similar object with one
Command
.
-
-
Alternative 2: Add only one
Module
object with oneAddCommand
-
Pros: Easier to implement and consistent across all commands.
-
Cons: More to type if user intends to add multiple
Module
objects.
-
Eventually, we decided on alternative 1 for the convenience of the user since the number of modules taken per semester is not low.
Aspect 2: How Deadline
feature is implemented
-
Alternative 1 (current choice): The
date
is compulsory whiletime
is optional for adeadline
-
Pros: Gives user the flexibility to input different types of tasks.
-
Cons: More bugs in
deadline
related method calls.
-
-
Alternative 2: Both
date
andtime
is compulsory for adeadline
-
Pros: Easier to implement since both date and time will be parsed.
-
Cons: Some tasks do not have a timing that it must be completed by, making it not user-friendly.
-
Eventually, we decided on alternative 1 for the benefits of the user. To tackle the cons, we have set time
to be "23:59" by default if user did not specify.