Overview
MODdy is a desktop application used by NUS Computing students to track their course progression, manage modules and manage deadlines. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 10 kLoC.
Summary of contributions
-
Major enhancement: added the ability to create a new profile
-
What it does: Allows the user to create his/her profile, which includes his/her name, course, current semester and focus area.
-
Justification: This feature improves the product significantly because it is what makes the app personal, and all the data such as modules and tasks of the user will be stored in the profile created using this command.
-
Highlights: There were many design considerations involved, such as ways to restrict user input to valid courses and focus areas, as well as finding an effective way to store all the user’s modules, grades and deadlines within the profile.
-
-
Major enhancement: added the ability to add modules and tasks
-
What it does: Allows the user to add NUS modules to a specific semester, whereby each module can store the user’s grades as well as a list of deadlines which can be added at any time by the user.
-
Justification: This is the main feature of the product as it allows the user to add modules and other information that he/she wants to add into the product.
-
Highlights: There were many design considerations involved, for example, which fields in the user input should be compulsory and how the information should be stored within the module. Additionally, since the user is allowed to add both a new module and a new deadline task to an existing module, there had to be considerations as to how to differentiate between them using the same command.
-
-
Major enhancement: added the ability to edit profiles, modules and tasks
-
What it does: Allows the user to edit his/her user profile, edit module details of a module that was added previously and edit the task description and deadline of a deadline task added to a module previously.
-
Justification: This feature improves the product significantly because the user can edit any element of his profile, module and tasks, without having to delete it and add it again. This gives the user much more flexibility in the usage of the product.
-
Highlights: There were many design considerations involved, such as which elements should be editable to make the product user-friendly. Additionally, there were considerations as to how to specify which module/task is meant to be edited.
-
-
Minor enhancement: added the ability to check that the user’s course is a valid computing course and that his/her focus area is valid for the specified course.
-
Code contributed: [Functional and test code]
-
Other contributions:
-
Enhancements to existing features:
-
Documentation:
-
Wrote the About Us and Contact Us documentation (Pull request #19)
-
Contributed the
edit
command documentation in the user guide -
Contributed segments to the developer guide such as logic, dependency between modules and personal classes as well as the edit command
-
-
Community:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Editing profile, module or task: edit
If you want to edit any of the attributes you have previously added to MODdy, you can do so by using the edit
command to edit your profile, module details or deadline tasks.
You don’t have to worry about adding any wrong information as you can edit them at any time!
There are three ways you can use the edit
command:
Editing your profile
Format: edit [n/name] [c/course] [y/year.semester] [f/focusArea]
Example: edit n/Brad c/Information Systems
edits your profile name to "Brad" and your course to "Information Systems",
as shown in Figure 8 below.
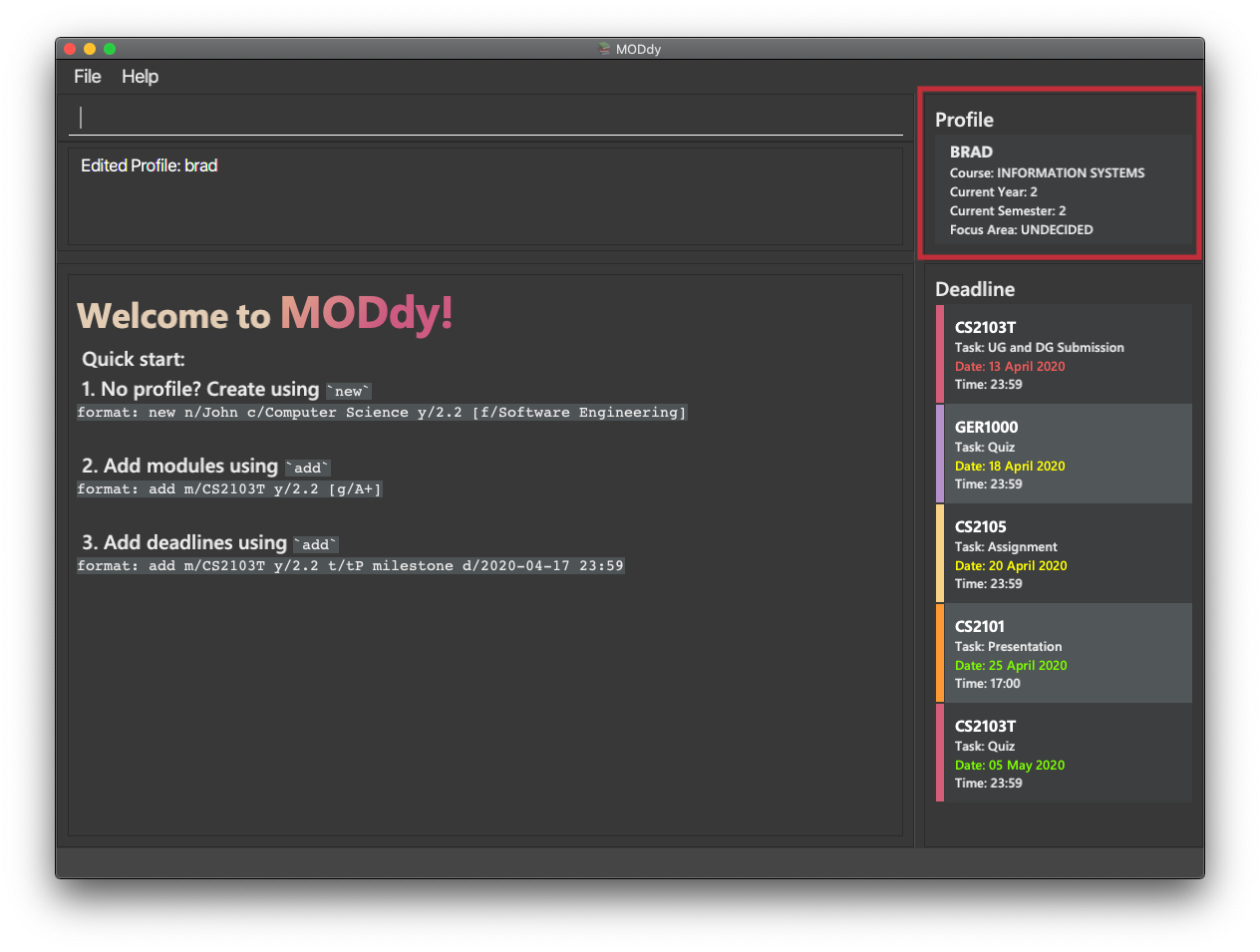
edit n/Brad c/Information Systems f/Electronic Commerce Alongside your name and course, you can edit your focus area to match your current course using this command. |
Editing a module’s details in MODdy
Format: edit m/moduleCode [y/year.semester] [g/grade]
Example: edit m/CS2103T g/A+
edits your grade of the module CS2103T to A+, as shown in Figure 9 below.
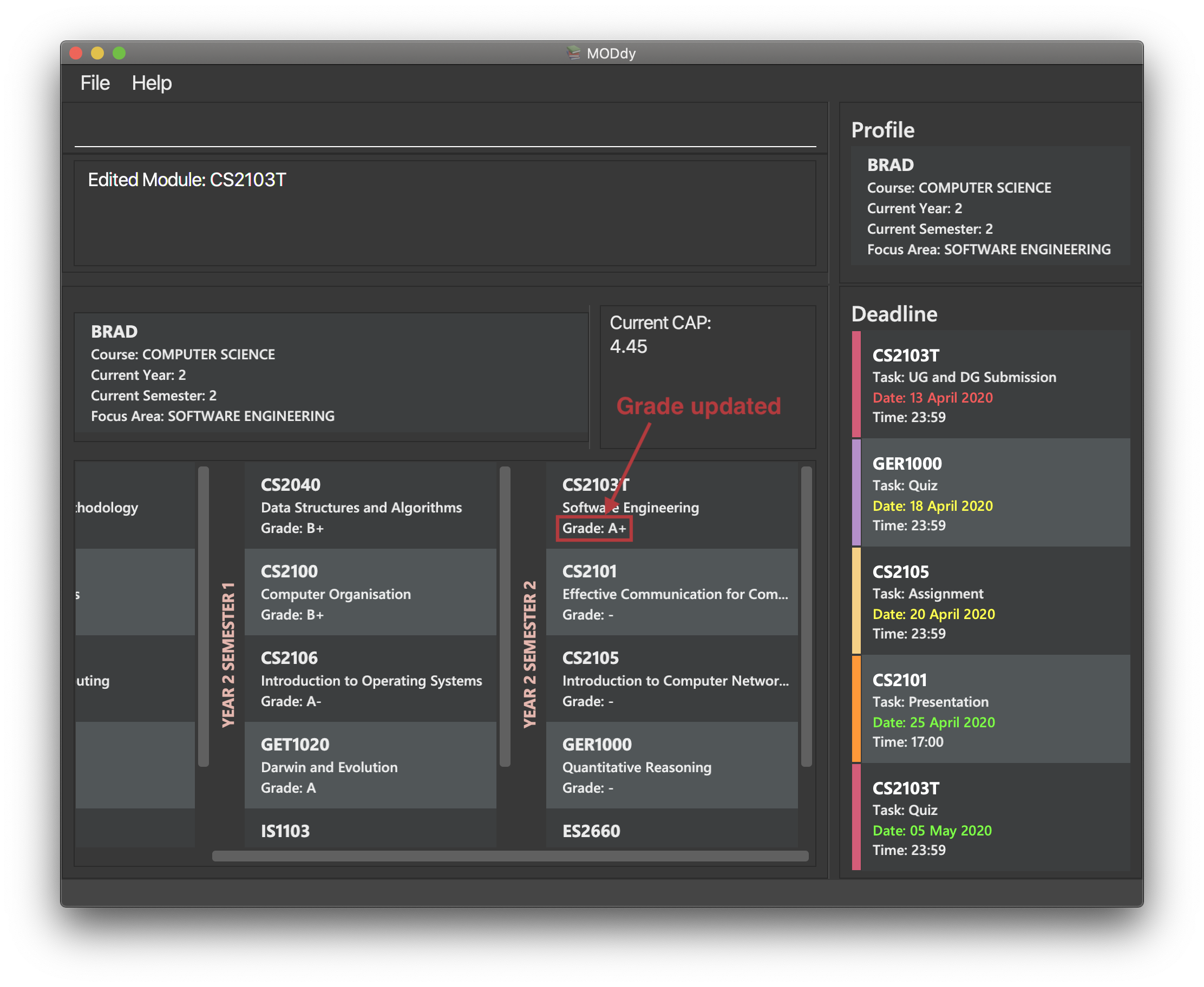
edit m/CS2103T y/2.1 g/A+ You can edit the grade of module CS2103T to A+ and change the semester which the module is taken in to Year 2 Semester 1 concurrently. |
If you edit a module in your current semester to another semester, all tasks associated to this module will be removed from the deadline panel. To bring it back, edit the module’s semester back to your current semester. |
Editing a task’s description or deadline
Format: edit m/moduleCode t/task [nt/newTask] [d/deadline]
Example: edit m/CS2103T t/tP Submission nt/UG and DG Submission
edits an existing CS2103T task "tP Submission" to new task name "UG and DG Submission", as shown in Figure 10 below.
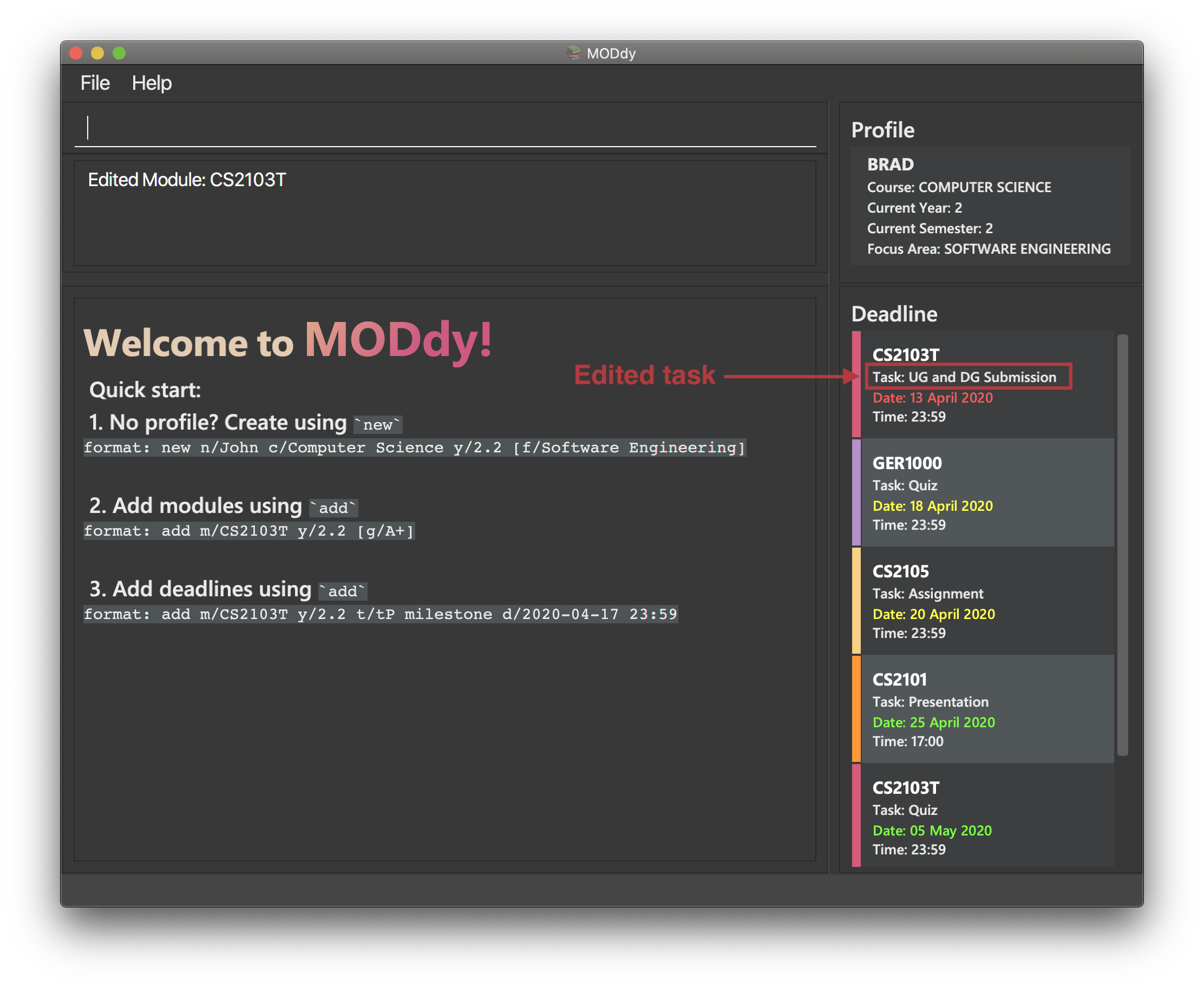
edit m/CS2105 t/Assignment d/2020-12-12 12:00 You can use this command to edit the deadline of Assignment, under module CS2105, to 12 December 2020 12:00. |
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Logic component
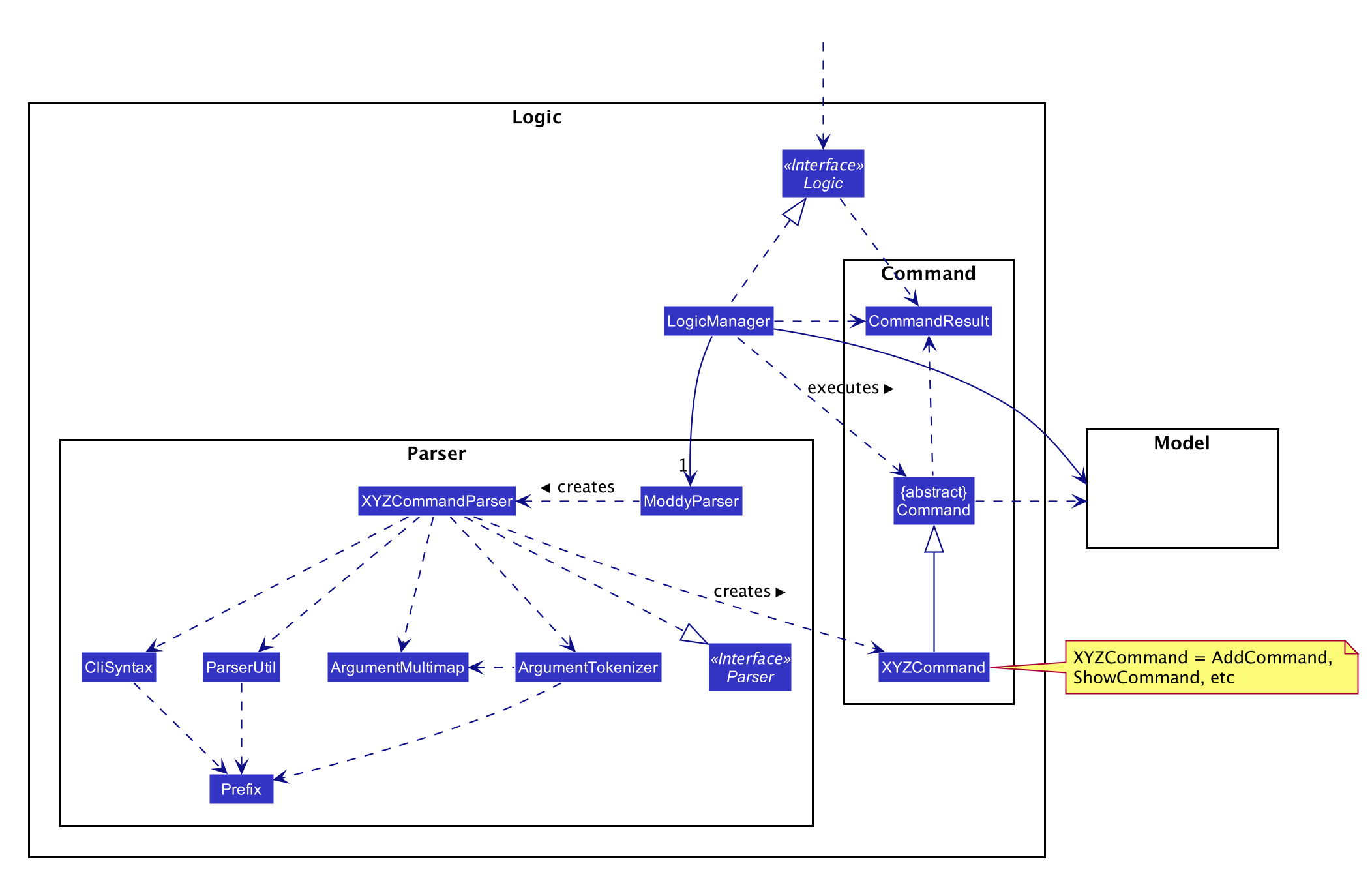
API :
Logic.java
-
Logic
uses theModdyParser
class to parse the user command. -
This results in a
Command
object which is executed by theLogicManager
. -
The command execution can affect the
Model
(e.g. adding aModule
). -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
. -
In addition, the
CommandResult
object can also instruct theUi
to perform certain actions, such as displaying help to the user.
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("delete m/CS2101 t/work")
API call.
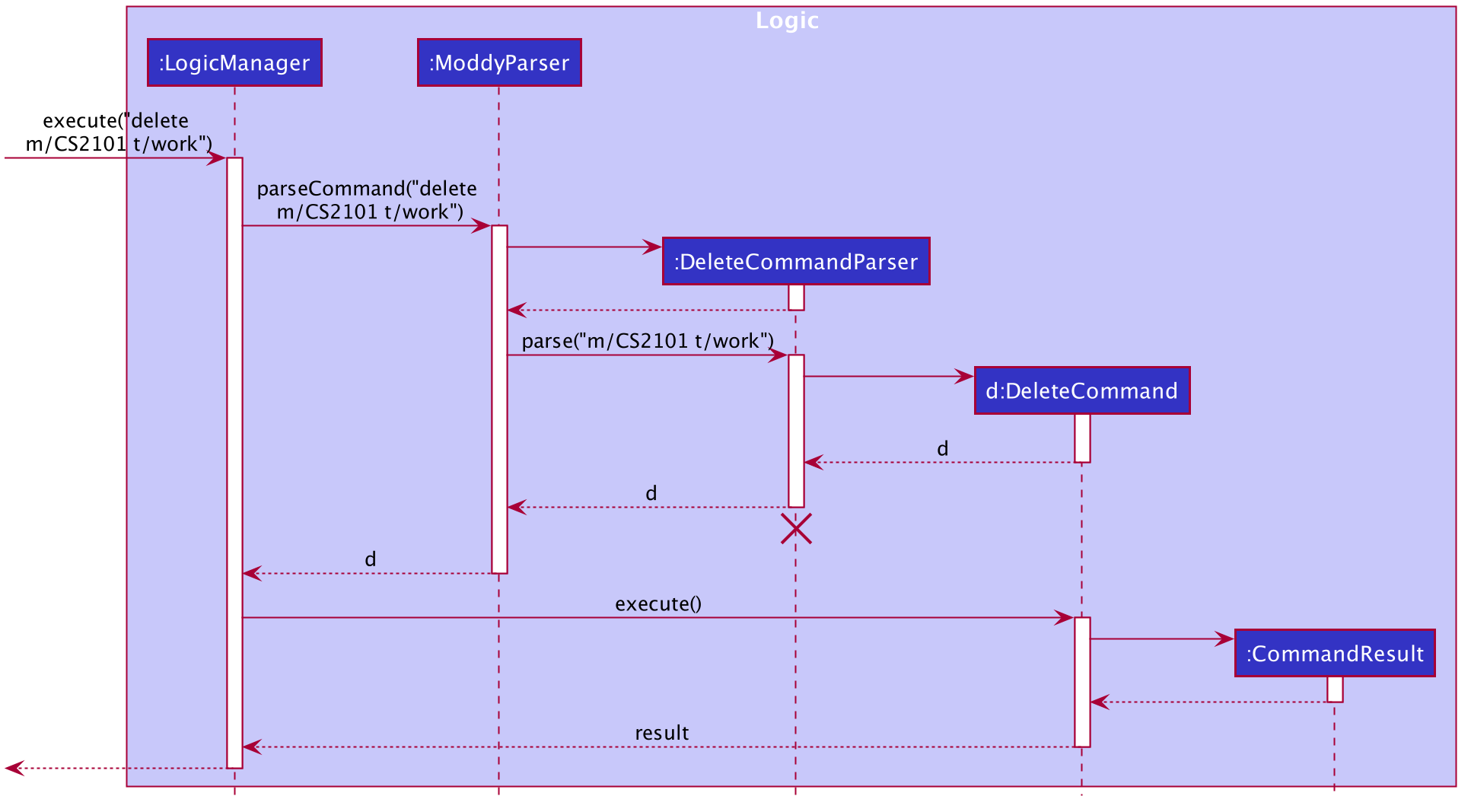
delete m/CS2101 t/work
Command
The lifeline for DeleteCommandParser should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of diagram.
|
Dependency between Module and Personal
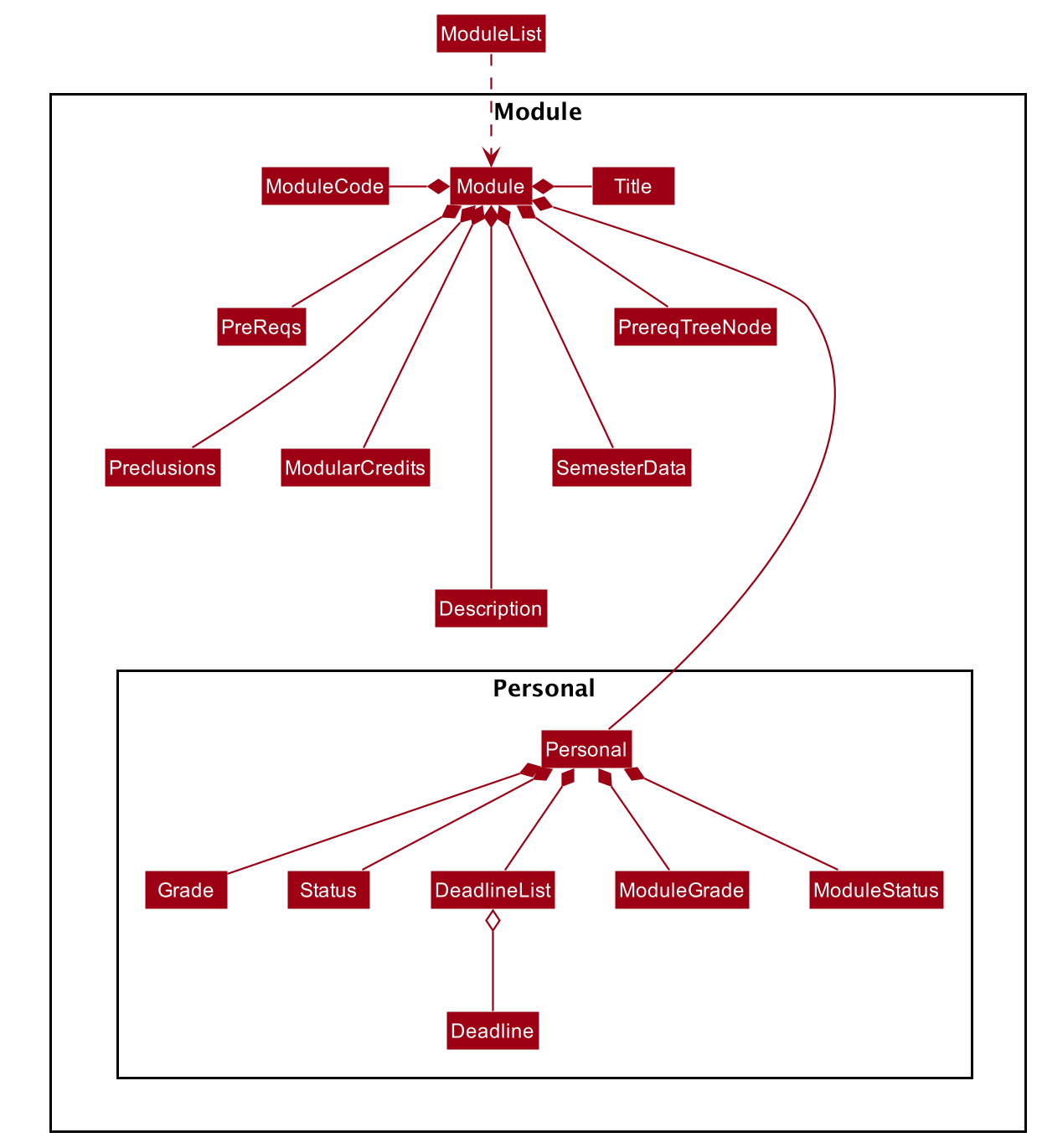
Each Module
class consists of a Personal
class, alongside other module attributes from NUSMODS. In each Personal
class, there are 3 attributes that are for the user to store their data:
-
Grade
: the user’s grade for the particular module -
Status
: the status of the module (COMPLETED, IN_PROGRESS, PLANNING) -
DeadlineList
: a list ofDeadline
tasks added by the user
These attributes were included in Personal
as they are the attributes that are personal to the user, and which the user can edit at any time.
ModuleGrade
and ModuleStatus
are enumeration classes that store valid grades and module statuses.
Edit Profile / Module Feature
The edit
feature supports the editing of both Profile
and Module
. Profile
is edited with the command edit
and Module
is edited with the command edit
appended with m/moduleCode
.
The edit profile feature is a complementary feature to the new profile feature. It allows the user to edit their profile that was created at the start. Profile features such as name, course, current year/semester and focus area can be edited using this command.
In addition, the edit module feature is a complementary feature to the add module feature. It allows the user to edit a module that has previously been added. The user can edit module information such as the semester it is taken and grade.
Lastly, the edit deadline feature is a complementary feature to the add deadline feature. It allows the user to edit a deadline that has previously been added to a module. The user can edit deadline information such as its description, date and time.
To edit the profile, the command edit
should be appended with one or more of the tags:
-
n/name
: New name -
c/course
: New course -
y/year.semester
: New current year/semester -
f/focusArea
: New focus area
To edit a module, the command edit
should be appended with m/moduleCode
, followed by one or more of the tags:
-
y/year.semester
: New year/semester where module is taken -
g/grade
: New grade for the module -
t/task
: Old task description-
nt/newTask
: New task description -
d/deadline
: New date and time
-
To edit a deadline that has previously been added, append edit m/moduleCode
with t/task
which is the description of the task to be edited, followed by either nt/newTask
which is the new description of the task and/or d/deadline
which is the new deadline that will replace the existing one.
Current Implementations
EditCommand
extends from the Command
class and uses the inheritance to facilitate the implementation. EditCommannd
is parsed using EditCommandParser
to split the user input into relevant fields.
The following sequence diagram shows how the edit
command works: edit n/John
:
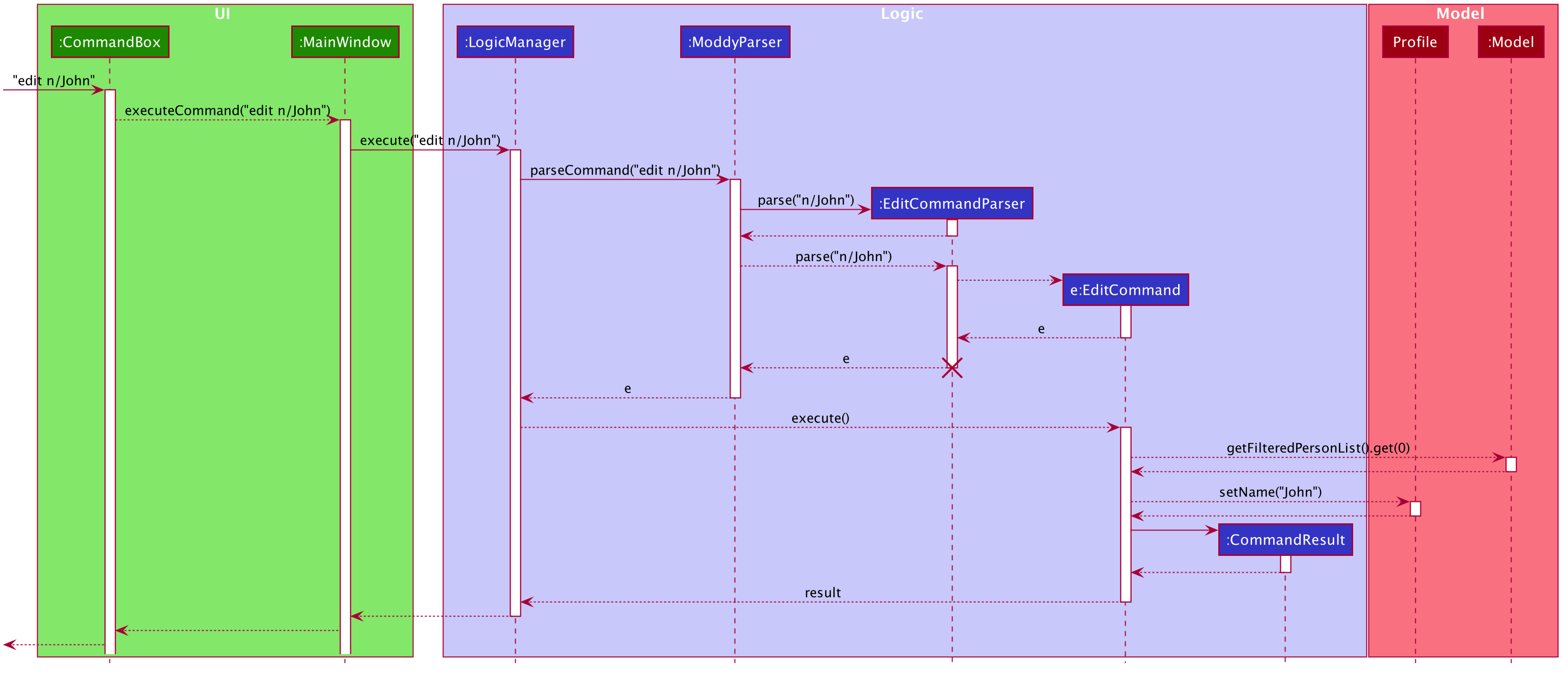
edit n/John
CommandDesign Considerations
-
Alternative 1 (current choice): Edit
Profile
,Module
orDeadline
with oneEditCommand
-
Pros: Easier for the user as they will not have to remember different edit commands for different purposes.
-
Cons: More considerations to differentiate the intent of the user and there is more room for error.
-
-
Alternative 2: Have separate commands to edit
Profile
,Module
andDeadline
-
Pros: Makes it clearer what the user intent is and is easier to parse.
-
Cons: Harder for the user to remember the commands and the user will not be allowed to edit both module details and deadline tasks within the module at the same time.
-
Eventually, we decided on alternative 1 due to the benefits of consistency. Additionally, by requiring a m/moduleCode
field for the user to edit Module
, it clearly shows the intent of the user, and whether the Profile
or a Module
should be edited.
We also considered whether to allow users to edit the grade of a module that has yet to be taken. We eventually decided to allow that as many students may want to predict their CAP, which our CAP calculator will allow them to do.
The following activity diagram shows how the edit
command decides what to edit (profile, module details or deadline task):
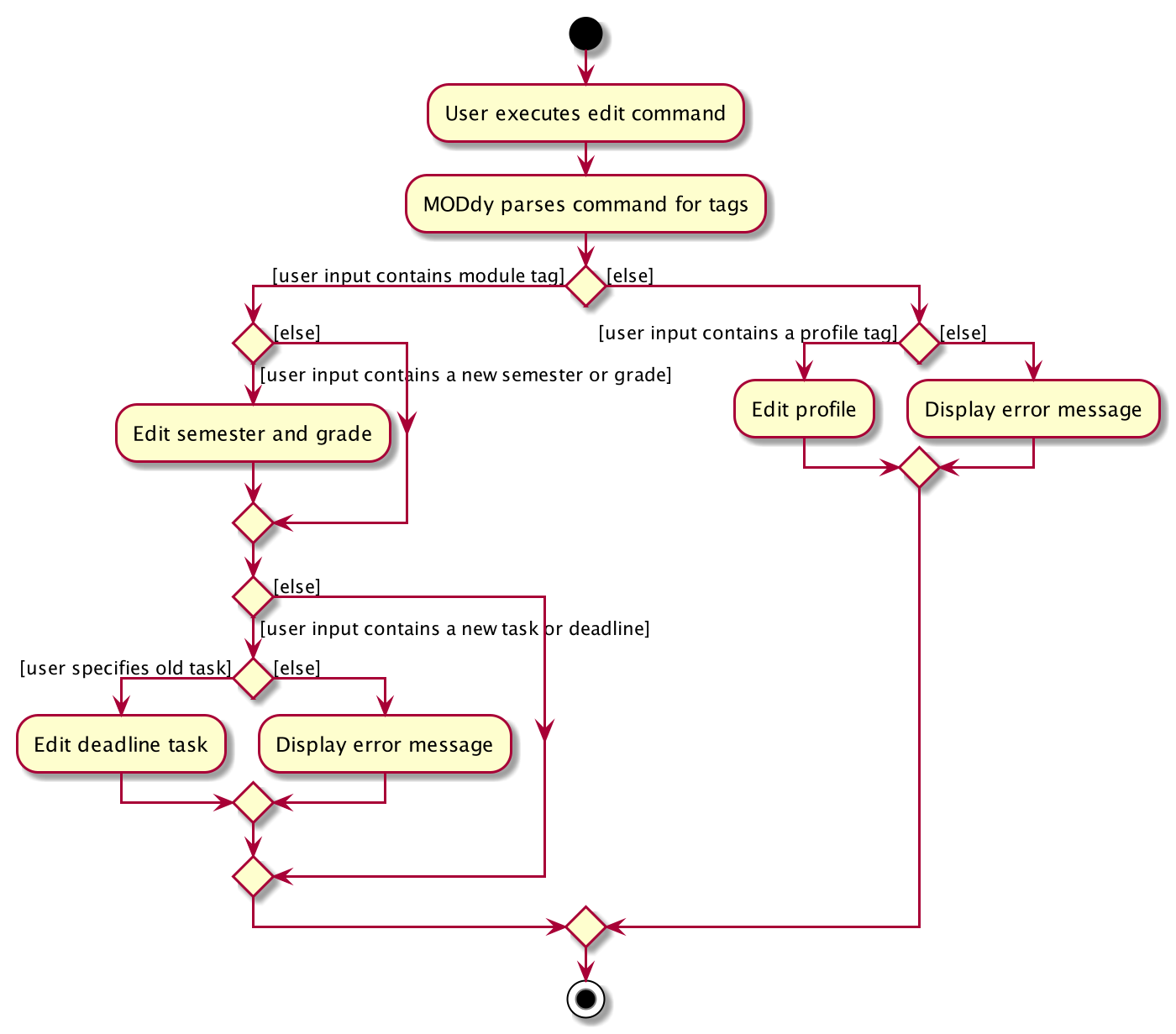