Overview
MODdy is a desktop application used by NUS Computing students to track their course progression, manage modules and manage deadlines. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 10 kLoC.
Summary of contributions
-
Major enhancement: Refactored all commands to take in Manager objects
-
What it does: Allows developers and commands to have non-static access to Manager objects
-
Justification: Previous usage of Manager object attributes were accessed using public static methods that did not align with good coding practices
-
Highlights: This enhancement prevents other classes from illegal and unintended access of Manager objects. It required a good understanding of what every command was doing and being able to change static variables to non-static ones.
-
-
Minor enhancements:
-
Added a show command that allows the user to display further details of course-related information in MODdy
-
Added the ability to store course information in JSON format that can be used by all users of MODdy
-
Added the feature to add deadlines to and delete deadlines from a module (Pull requests #321 #327)
-
Credits: With reference to @chanckben's JsonModule class
-
-
Added Cumulative Average Point (CAP) calculator for users to keep track of their grades
-
Ensured features were working as intended for each commit and version release. Raised and also solved multiple issues (Issues raised)
-
-
Code contributed: [RepoSense report]
-
Other contributions:
-
Project management:
-
Managed the production of MODdy by leading the discussion of its initial implementation
-
Managed the overall progress of MODdy by directing weekly team meetings
-
-
Enhancements to existing features:
-
Implemented Show command (Pull request #115)
-
Implemented Module class (Pull request #29)
-
Implemented CourseManager class and its JSON storage (Pull requests #94 #119)
-
Created CAP calculator (Pull request #155)
-
Refactored commands to non-static access of Manager objects (Pull request #151)
-
Addressed and closed numerous issues raised by other users in dry Practical Exam (Pull request #259)
-
-
Documentation:
-
Did cosmetic tweaks to existing contents of the User Guide: #280
-
-
Community:
-
Reported bugs and suggestions for other teams in the class (examples: 1)
-
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Showing profile, module, semester, course or focus area: show
Not all information can be seen at once. To see this information you have added previously, use the show
command to switch your current MODdy display.
There are five ways you can use the show
command:
Showing your profile
Format: show n/name
Example: show n/Brad
shows the profile overview of user "Brad", as shown in Figure 11 below.
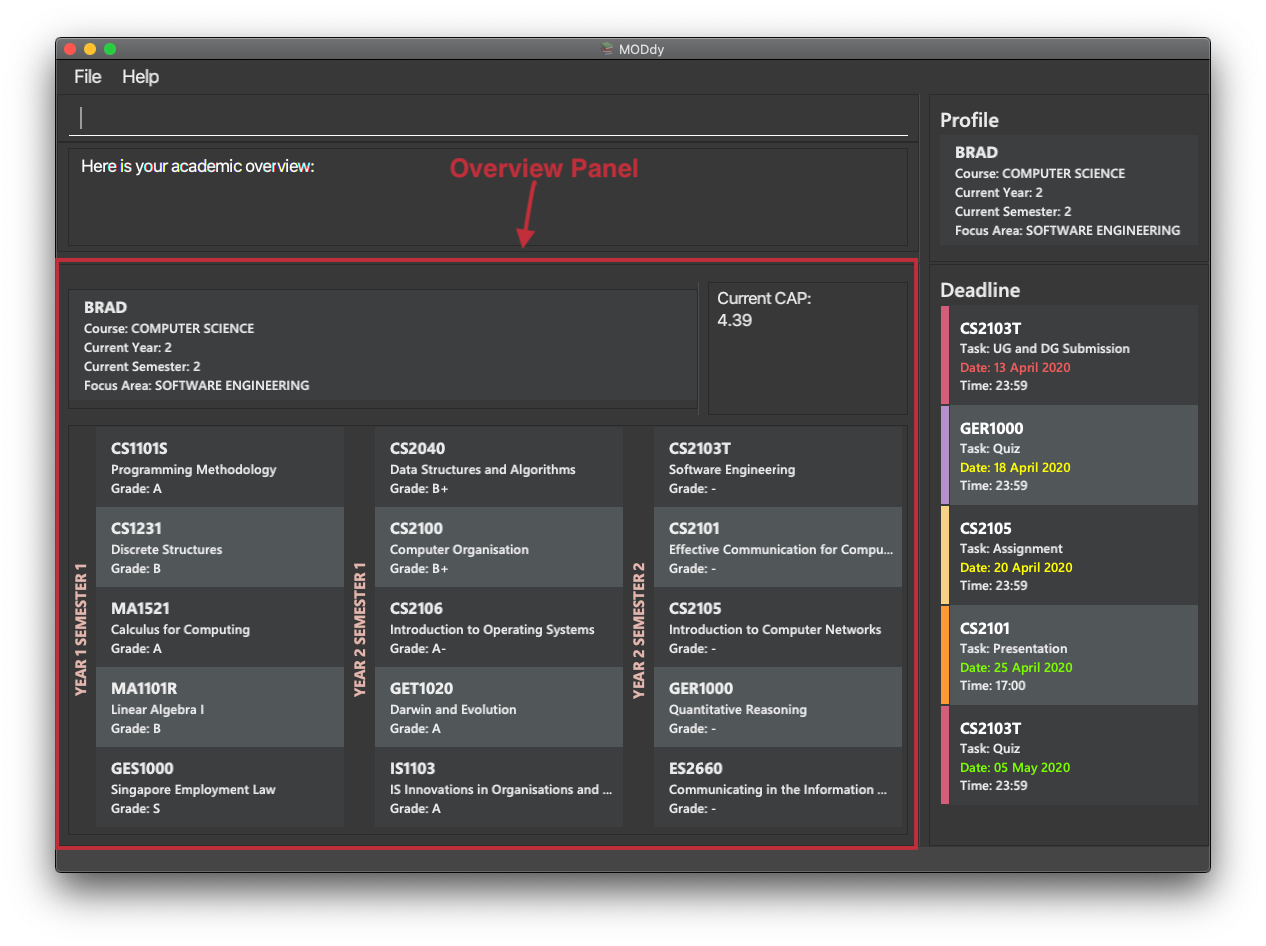
All the modules under every semester, their grades, as well as your current CAP will be displayed. |
Showing details of a module
Format: show m/moduleCode
Example: show m/CS2103T
show all the module details of CS2103T, as shown in Figure 12 below.
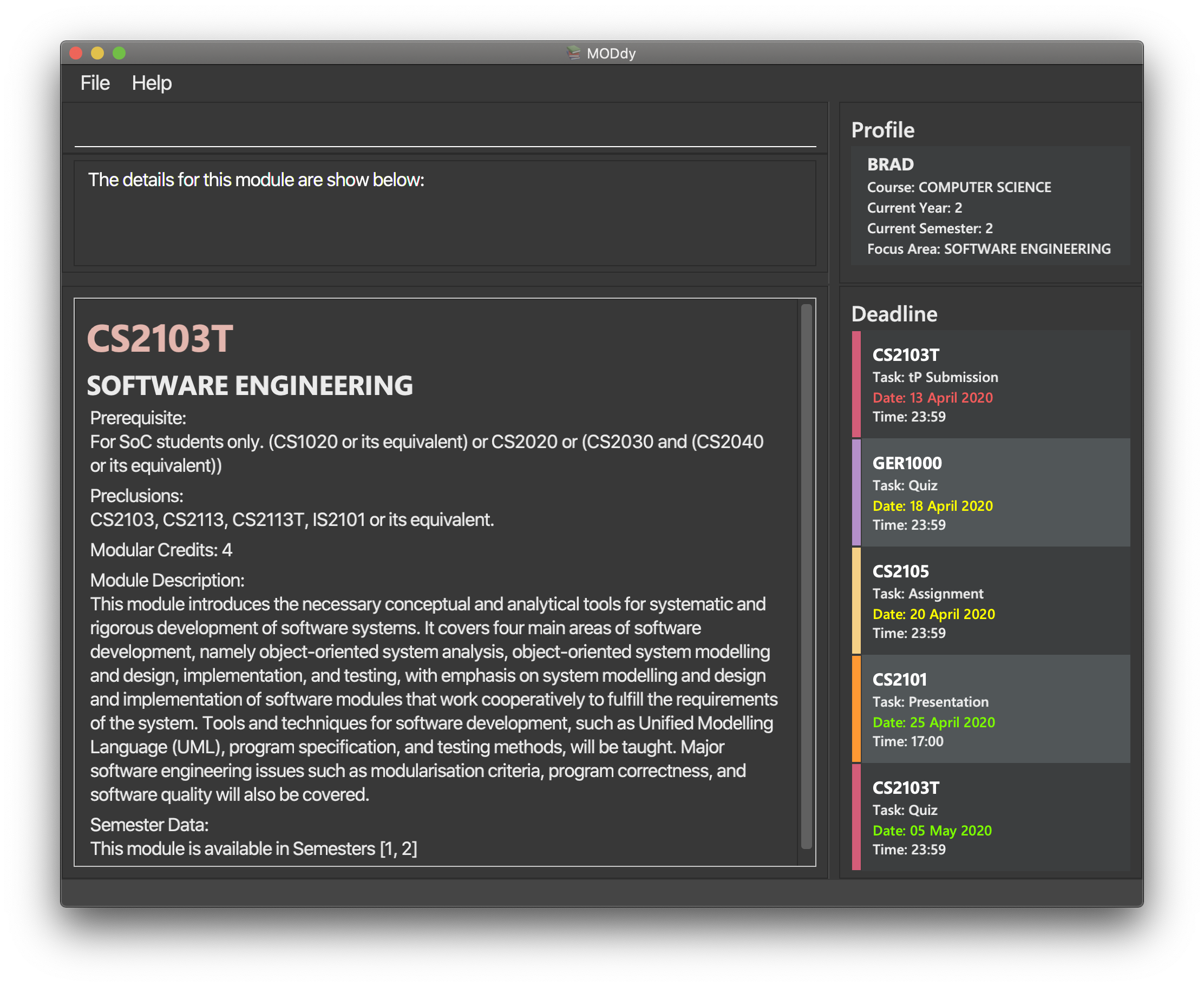
The module name, prerequisites, modular credits, description and semesters the module is offered in will be displayed. |
If you request for MODdy to show multiple information at one time, such as course information on Computer Science and module information on CS1101S, using the command show c/Computer Science m/CS1101S , no objects will be displayed.MODdy will remind you that you can only display one object at a time. |
Showing modules in the specified semester
Format: show y/year.semester
Example: show y/1.1
shows all modules taken in Year 1 Semester 1, as shown in Figure 13 below. If grades for these modules
are available, it will be displayed as well.
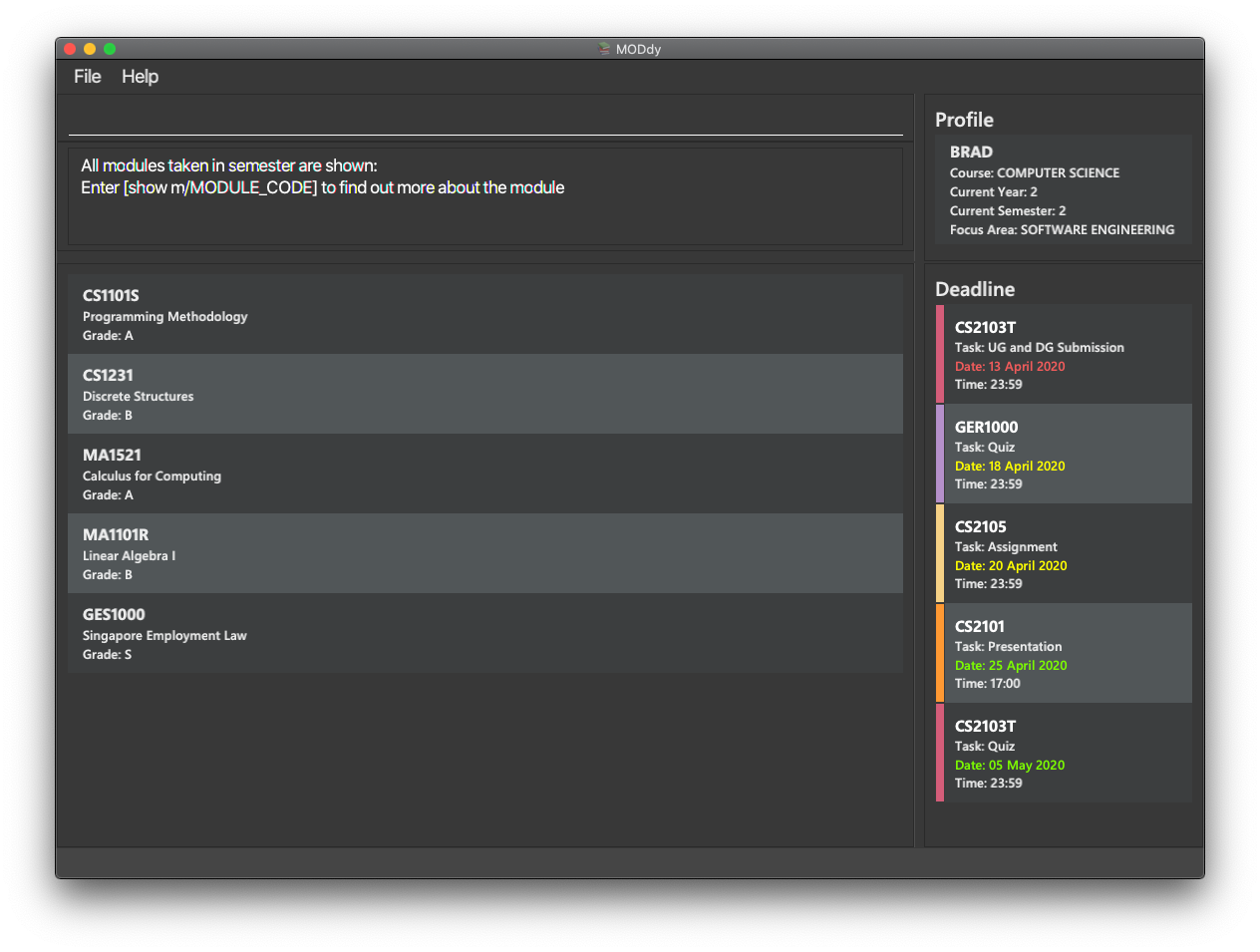
Showing the course’s requirements
Format: show c/course
Example: show c/Computer Science
shows the course requirements and focus areas of "Computer Science", as shown in Figure 14 below.
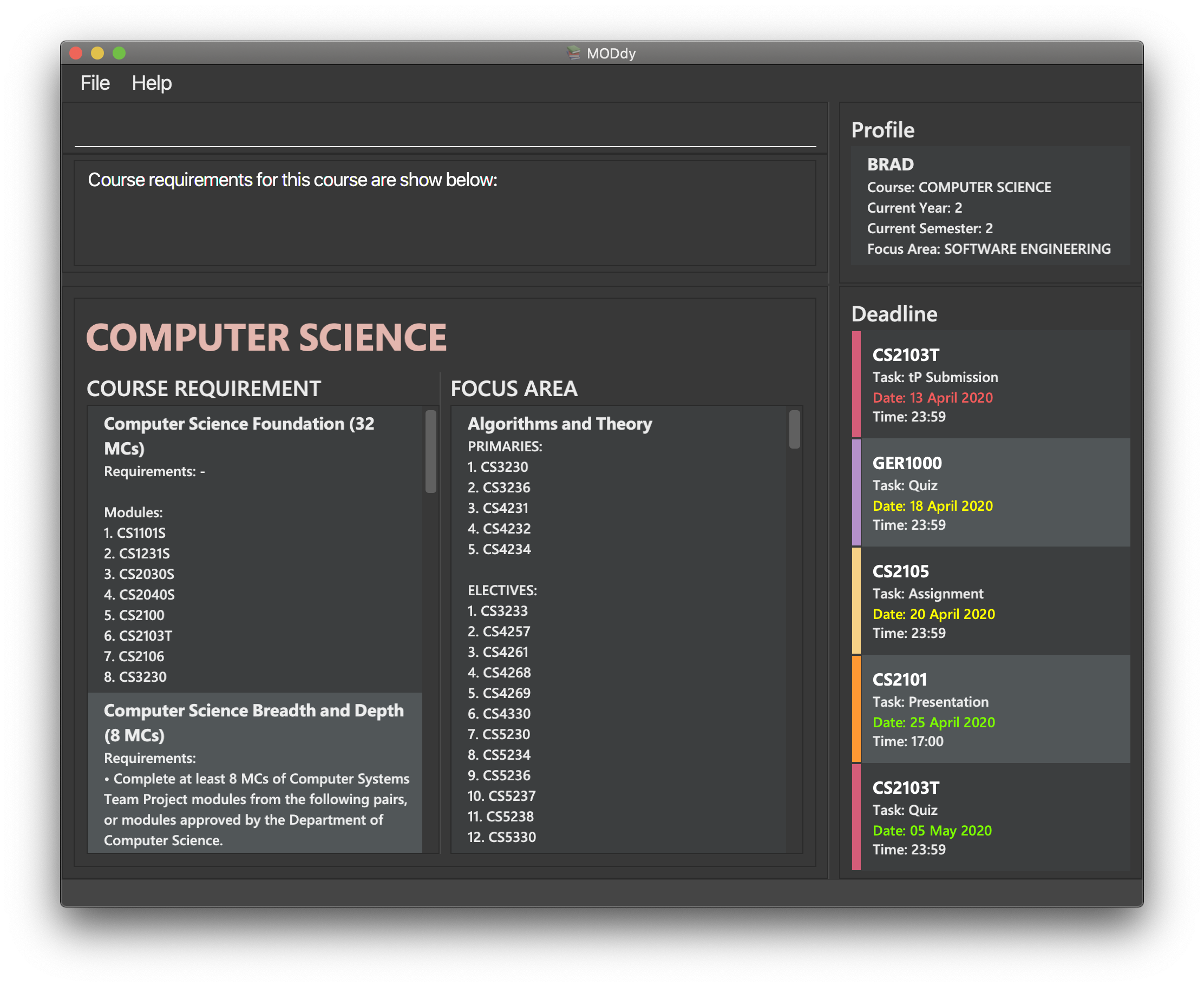
You need to enter the course name in full! |
Showing modules under the specified focus area
Format: show f/focusArea
Example: show f/Software Engineering
shows the Primaries and Electives of focus area "Software Engineering",
as shown in Figure 15 below.
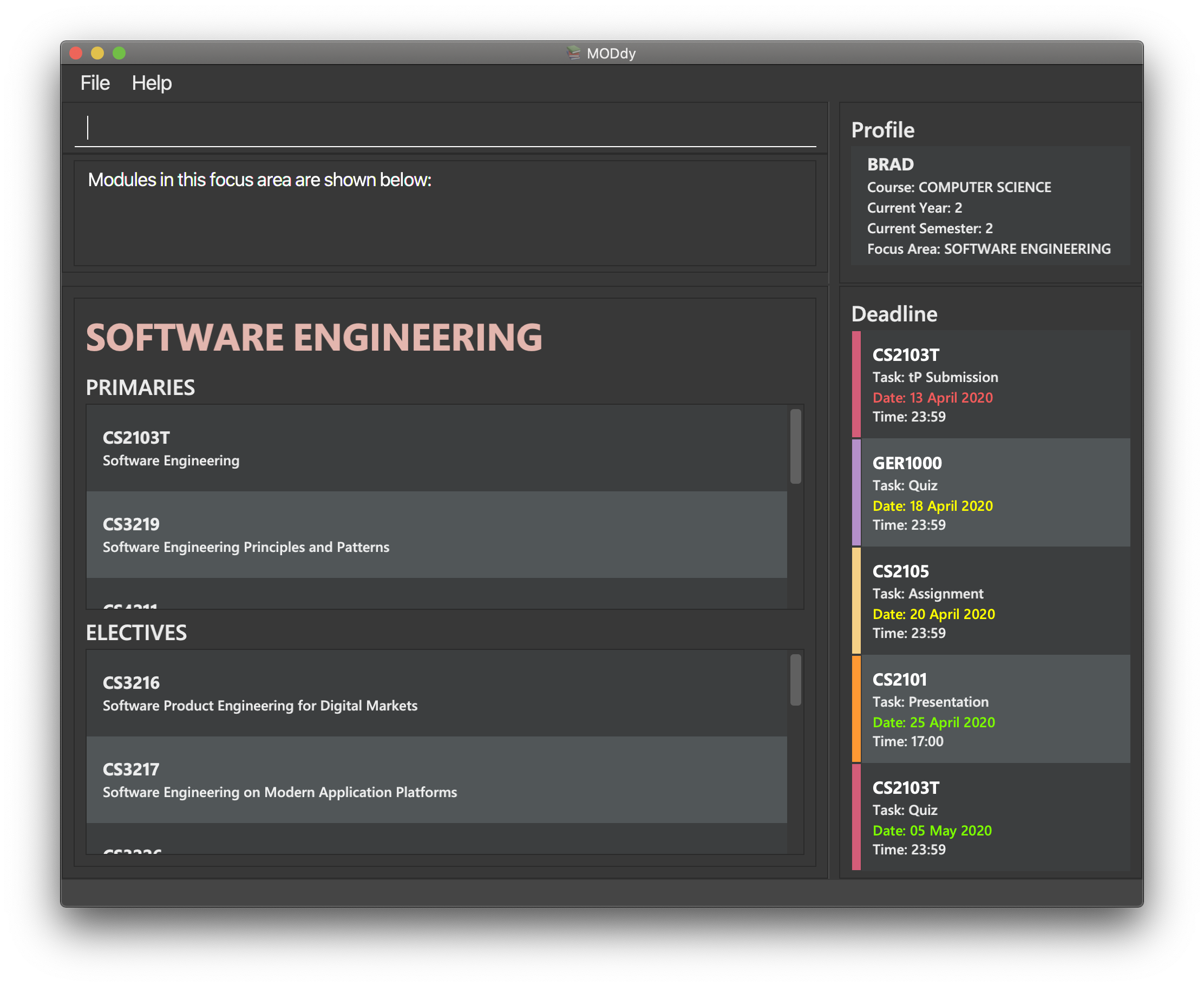
You can retrieve the list of focus areas under a course using the show c/course command. This list can also be found in the Appendix of this guide.
|
Appendix
-
List of courses and focus areas (if applicable) under each course currently supported by MODdy
-
Business Analytics
-
Financial Analytics
-
Marketing Analytics
-
-
Computer Engineering
-
Communications and Networking
-
Embedded Computing
-
Intelligent Systems
-
Interactive Digital Media
-
Large-Scale Computing
-
System-On-A-Chip Design
-
-
Computer Science
-
Algorithms and Theory
-
Artificial Intelligence
-
Computer Graphics and Games
-
Computer Security
-
Database Systems
-
Multimedia Information Retrieval
-
Networking and Distributed Systems
-
Parallel Computing
-
Programming Languages
-
Software Engineering
-
-
Information Security
-
Information Systems
-
Digital Innovation
-
Electronic Commerce
-
Financial Technology
-
-
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Model component
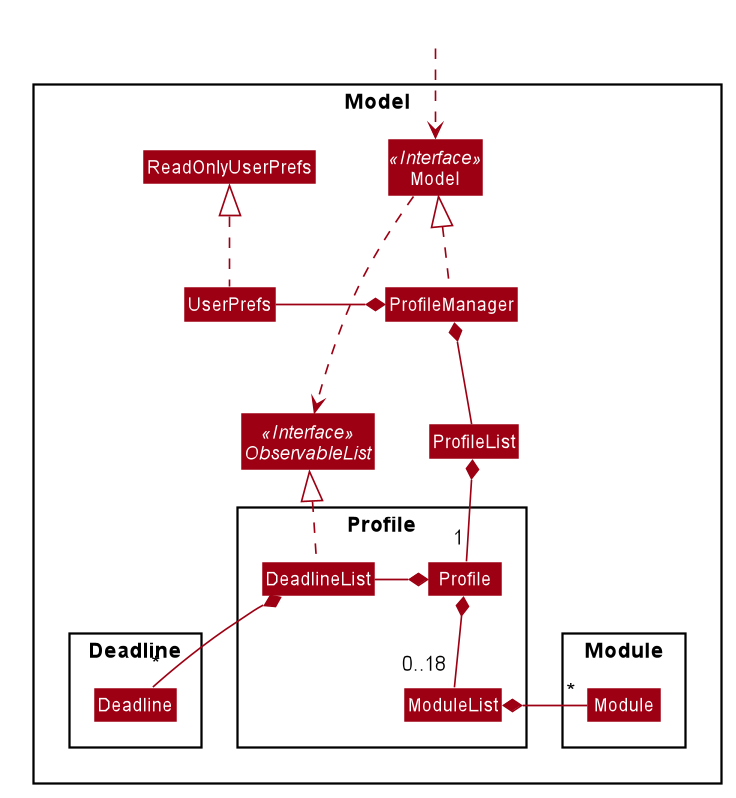
The Model
,
-
stores a
UserPref
object that represents the user’s preferences. -
stores the profile data.
-
stores a
ModuleList
of modules taken in each semester in aHashMap
-
exposes an unmodifiable
ObservableList<Deadline>
that can be 'observed' e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list changes. -
does not depend on any of the other three components.
Show Profile / Module / Semester / Course / Focus Area Feature
The show
feature allows the user to display information about a Profile
, Module
, Course
, FocusArea
or Semester
with the command show
, appended with the tags below. These information cannot be seen from the main UI and have to be displayed through the show
command.
The tags are:
-
n/name
forProfile
-
m/moduleCode
forModule
-
c/course
forCourse
-
f/focusArea
forFocusArea
-
y/year.semester
forSemester
Current Implementations
ShowCommand
extends from the Command
class and uses the inheritance to facilitate the implementation. ShowCommand
is parsed using ShowCommandParser
to split the user input into relevant fields.
The following sequence diagram shows how the show
command works: show n/John
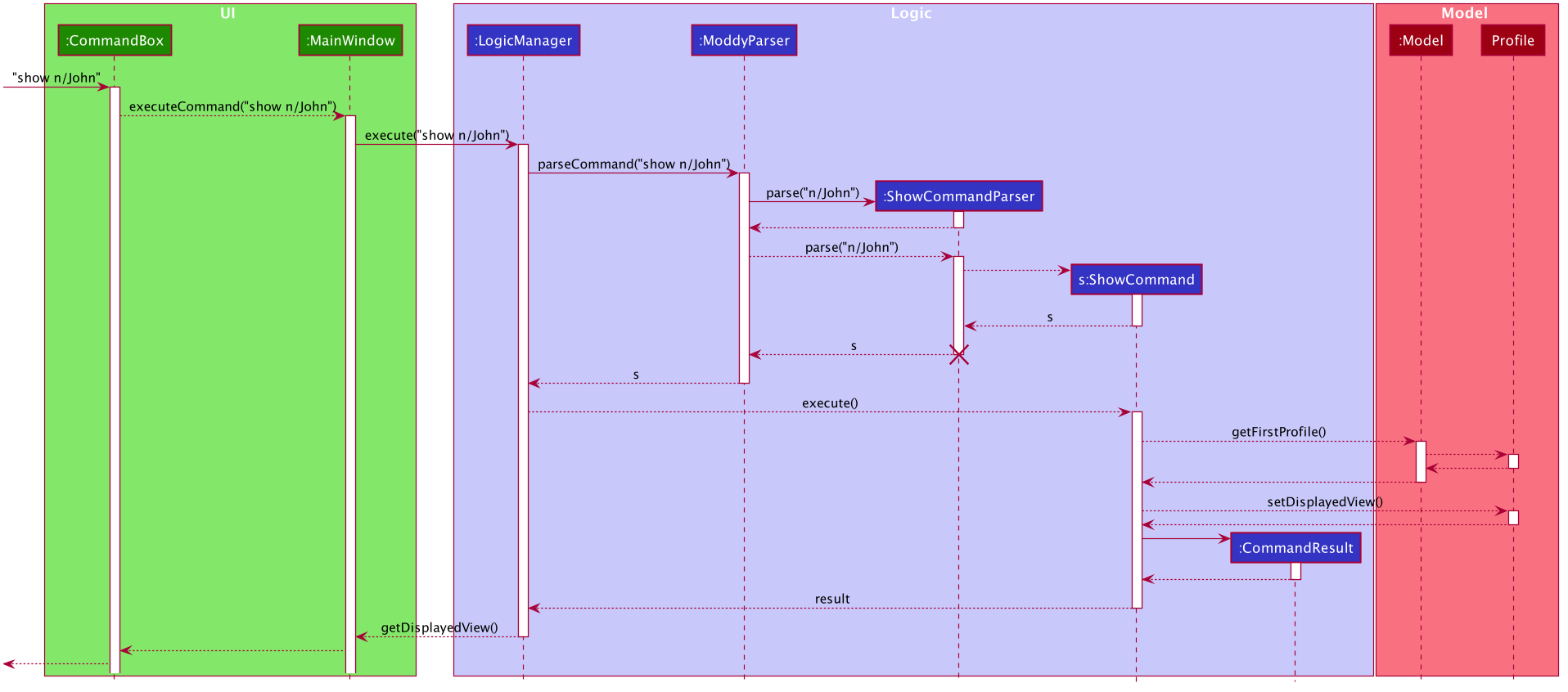
show n/John
commandThe following activity diagram shows how the show
operation works with input: show c/Computer Science
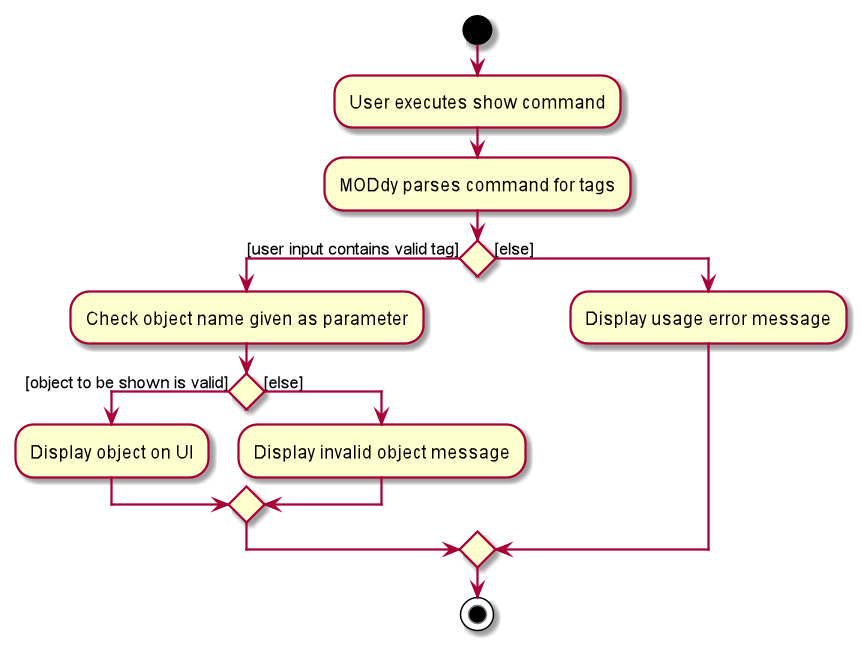
If more than one accepted tag is given, such as show c/Computer Science m/CS1101s , MODdy will not display anything and will instead give the user a message that only one tag should be provided.
|
Design Considerations
-
Alternative 1 (current choice): Have one
ShowCommand
for all objects to be displayed-
Pros: Repeated code is avoided
-
Cons: Takes in an
Object
in its constructor, anyObject
can call this method and cause unintended usages
-
-
Alternative 2: Have a separate
ShowCommand
(e.g.ShowModuleCommand
,ShowCourseCommand
) for each object shown-
Pros: Applies Single Level of Abstraction Principle (SLAP)
-
Cons: Too many classes having repeated code
-
Eventually, we decided on alternative 1 due to the benefits of avoiding repeated code. To tackle the cons from this, we implemented guard conditions to gracefully reject other objects that may unintentionally call this method.
Appendix A: Effort
In this section, we highlight the amount of effort took for us to develop MODdy, the challenges faced in this development and our eventual achievement.
Overall effort
Estimated effort: 16
The above estimated effort uses Address Book 3 (AB3) as a base estimation of 10 for effort taken.
In short, AB3 essentially stores data related to multiple persons. MODdy does that for one, but does much more. MODdy is able to store data for a user such as their course, focus areas and personal information of their modules, be it in the past, present or future.
There was a considerable amount of time spent throughout the construction of MODdy. We had to figure out how different courses and modules could be linked to each other in MODdy. We also had to plan out features that could allow users to make this course and module management application more personal. Features were not just implemented immediately, but significant considerations were taken to decide whether it was consistent with the other features that MODdy offered.
Feature implementation was also not just a single point of discussion. New features required edits to other features to maintain consistency, while some required even newer features to complement it. All these discussions were held at least once a week for a minimum of 2 hours each round to ensure that we were in sync with each others' work.
However, we also understand that AB3 has certain code classes that are complicated to build and these classes were also reused in MODdy. Examples of these reused classes are found in the commons
package, while MODdy-specific classes such as JsonSerializableCourseList
, JsonCourseListStorage
and JsonCourse
were implemented with heavy reference to original AB3 classes. Hence, the estimated effort was scaled back slightly.
Challenges faced
Ultimately, the biggest challenge was getting the User Interface (UI) of MODdy to be exactly as we envisioned. This UI was new and completely different from the existing AB3 UI, and this meant that we had to write entirely new code in an area which we were unfamiliar with. As a group of relatively inexperienced programmers, our prior application-building experiences were mostly limited to command-line interface applications. As such, implementing MODdy without any points of reference posed a huge challenge.
Another challenge was having to overcome issues and bugs that our new features brought. Manual testing took a significant amount of time, ensuring positive tests worked as they should, while we also had to design extensive negative tests to cover as many situations of undesired input as we could.
Achievements
MODdy turned out to be the best version of the MODdy that we had planned for in the first place. Initially, we scaled back on the number of features due to the difficulties faced during implementation, for fear of a lack of time. However, we were able to scale back up our efforts after overcoming difficulties and bugs faced, to bring back the initially planned features.
We are satisfied with the latest release version of MODdy as it is able to perform what we wanted it to do in the first place, to be a one-stop application for personal course and module management in NUS.