Overview
MODdy is a desktop application used by NUS Computing students to track their course progression, manage modules and manage deadlines. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 10 kLoC.
Summary of contributions
-
Major enhancement: added the ability to delete profiles, modules and tasks
-
What it does: Allows the user to delete his user profile, delete a module he has added previously, and delete a task which has been added to a module taken in the current semester.
-
Justification: This feature improves the product significantly because a user can remove a task after he has completed it, or remove a module if he decides to not take or drop the module, or remove his profile to reset MODdy.
-
Highlights: This enhancement is complementary to the feature of adding a new user profiles, modules and deadlines. There were many design considerations involved, such as the types of user inputs to accept, as well as whether to allow the user to delete multiple modules and tasks at once.
-
-
Major enhancement: implemented backend to enable MODdy to read data from and save data to relevant json files
-
What it does: Allows MODdy to parse module and user profile data from a json file into Java objects. Provides programmers with helpful classes to use the data stored in these objects. For user profile data, this enhancement also allows saving from Java object to json.
-
Justification: This feature improves the product significantly because modules and user profiles do not have to be manually added every time the application is loaded. The module and user profile data are stored in persistent storage. This reduces the risk of data loss for the user in the event of a system crash or power loss.
-
Highlights: This enhancement required pulling data from NUSMods using their API and cleaning up the data. The main challenge was deciding which fields of the data to keep or omit by thinking about their relevance to the users.
-
Credits: The ideas in the backend code of Address Book were reused and adapted to fit the code in this project.
-
-
Minor enhancement: added the ability to check if the user has fulfilled the prerequisites of a module prior to the semester the module is being added to.
-
Code contributed: Functional and test code
-
Other contributions:
-
Project management:
-
Set up the GitHub organisation and team repo
-
-
Enhancements to existing features:
-
Community:
-
Tools:
-
Integrated a new Github plugin (TravisCI) to the team repo
-
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Deleting profile, module, task or grade: delete
To remove a profile, module, task or grade from MODdy, use the delete
command.
There are four ways you can use the delete
command:
Deleting your profile
If you wish to remove your profile, you can do so with the command below. The effect of using this command is also shown in the figure below.
Format: delete n/name
Example: delete n/Brad
deletes "Brad" from the profile panel as well as all other data, as shown in Figure 16 below.
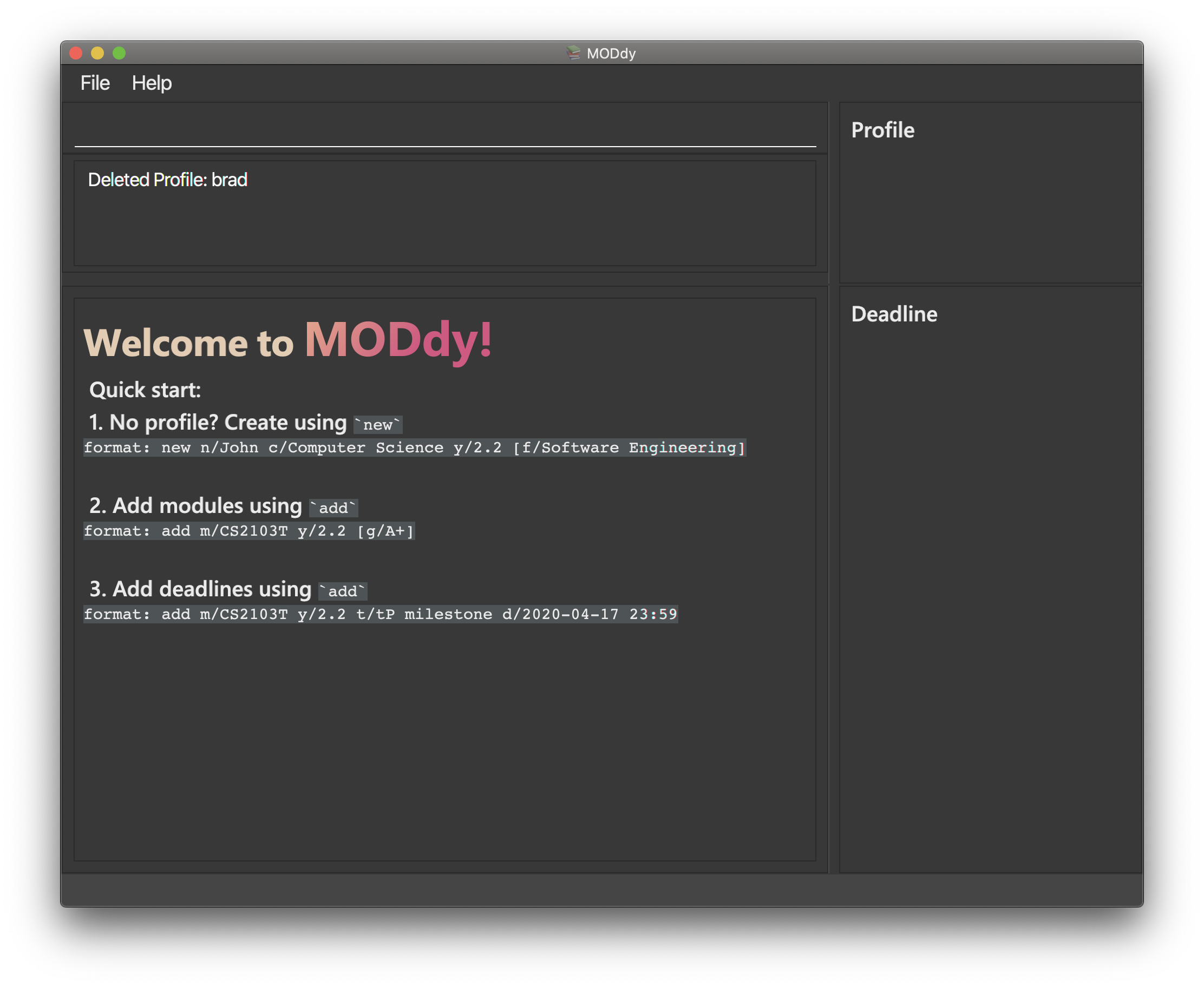
Your profile, including all modules, grades and deadlines under your name, will be deleted from MODdy |
Deleting a module
If you have decided not to take a module you were planning to take, or to drop a module which you are currently taking, you can delete it with the command below. The effect of using this command is shown in the figure below.
Format: delete m/moduleCode
You can also delete multiple modules at the same time. To delete multiple modules, just append the m/moduleCode
tags one after another, e.g. delete m/CS1231 m/IS1103 m/MA1521
.
Example: delete m/CS2103T
deletes CS2103T from Year 2 Semester 2 and also removes all tasks related to CS2103T from the deadline panel, as
shown in Figure 17 below.
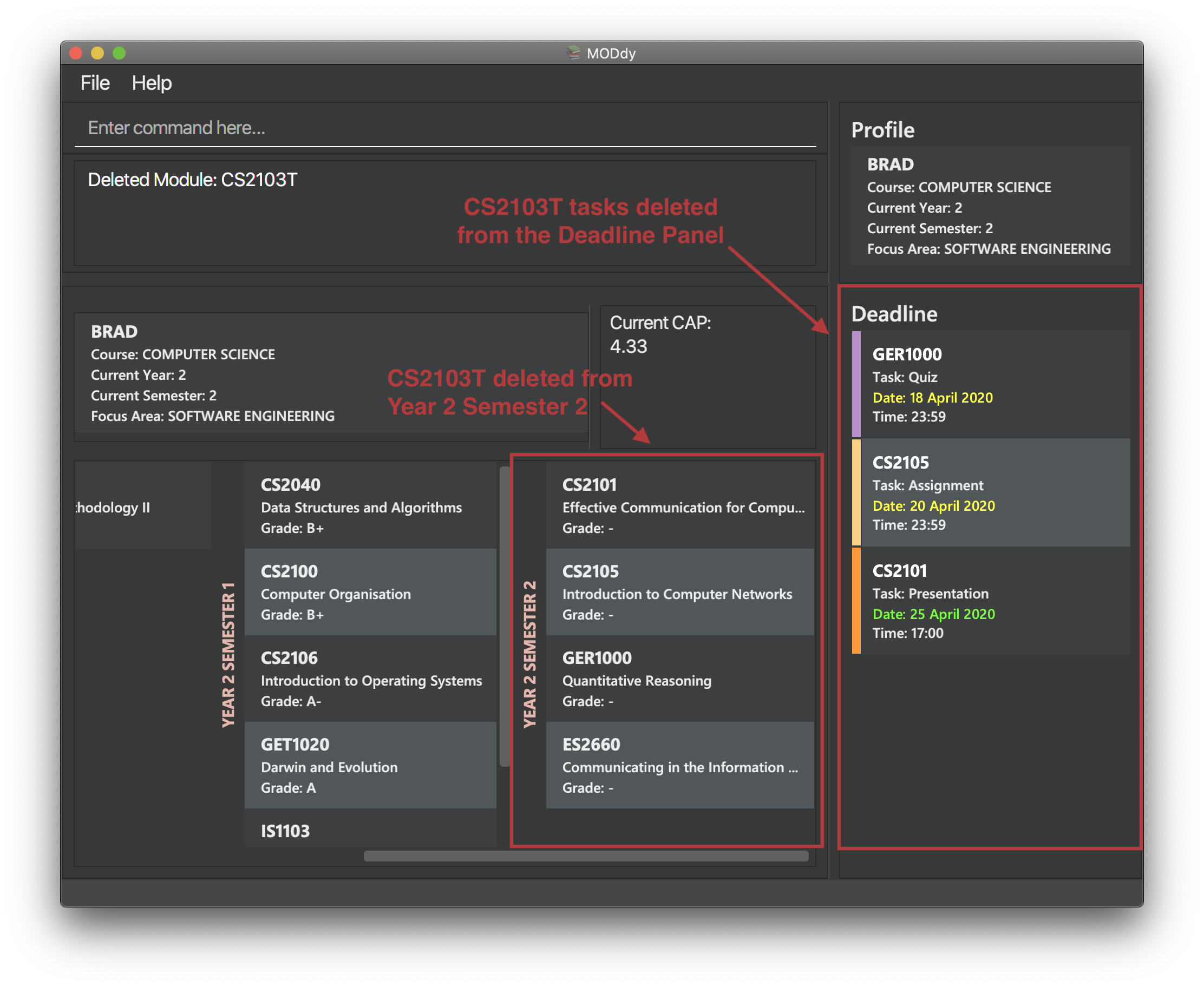
The specified module, including all tasks and deadlines of that module, will be deleted from MODdy. |
Deleting a task
Once you have completed a task, you can delete it using the command below. The effect of using this command is shown in the figure below.
Format: delete m/moduleCode t/task
You can delete multiple tasks at the same time but only from the same module. To delete multiple tasks, just append the t/task tags one after another, e.g. delete m/CS1231 t/quiz t/exam .
|
Example: delete m/CS2103T t/Quiz
deletes the task "Quiz" of module CS2103T from the Deadline Panel, as shown in Figure 18 below.
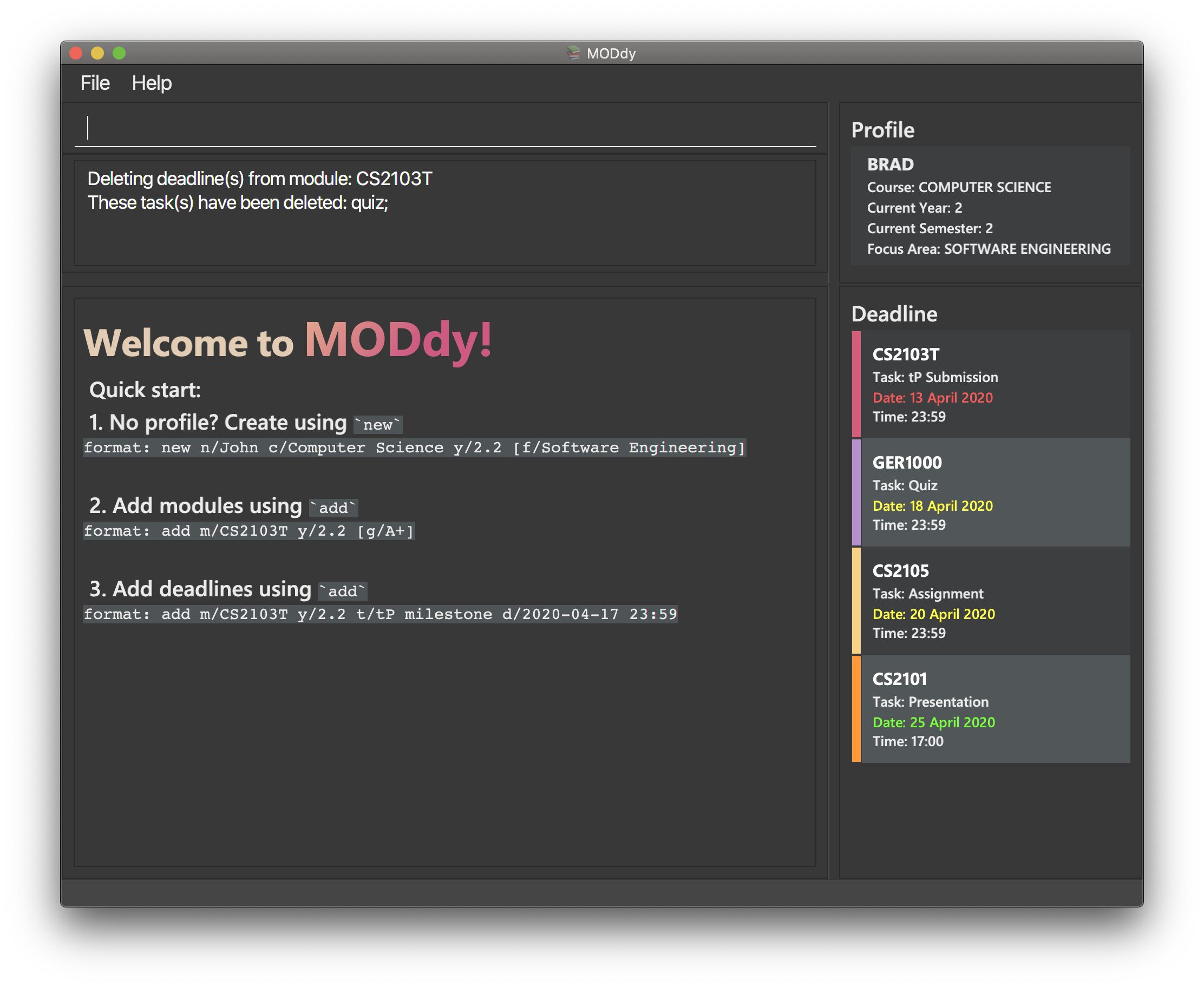
The specified task and its deadline will be deleted from the specified module. |
Deleting a grade
If you have been using MODdy to simulate your grades or you have entered a grade for the wrong module, you can delete the grade using the command below. The effect of using this command is shown in the figure below.
Format: delete m/moduleCode g/
Example: delete m/CS2103T g/
deletes the grade of module CS2103T, as shown in Figure 19 below.
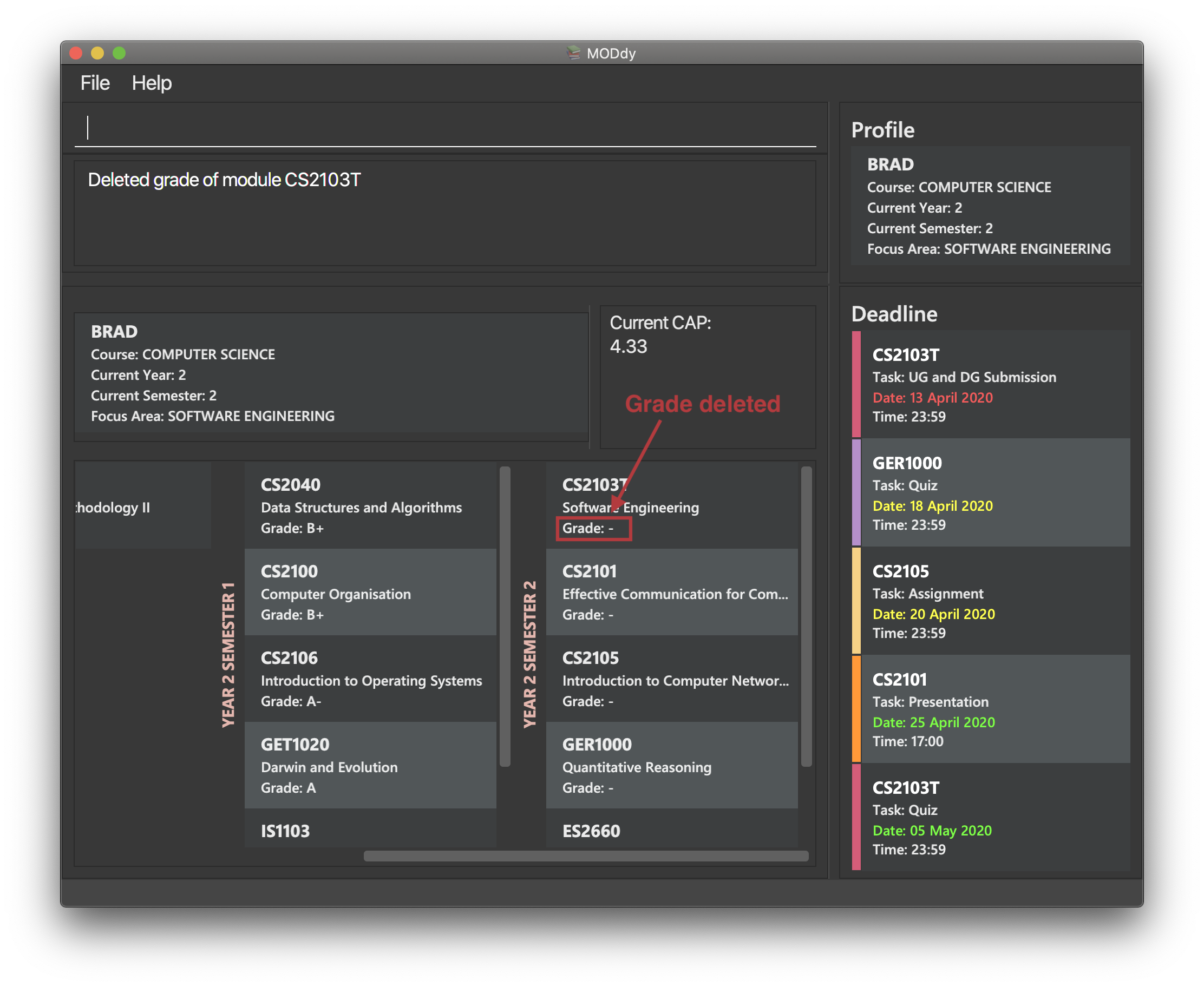
Any input after the g/ tag will be ignored.
|
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Storage component
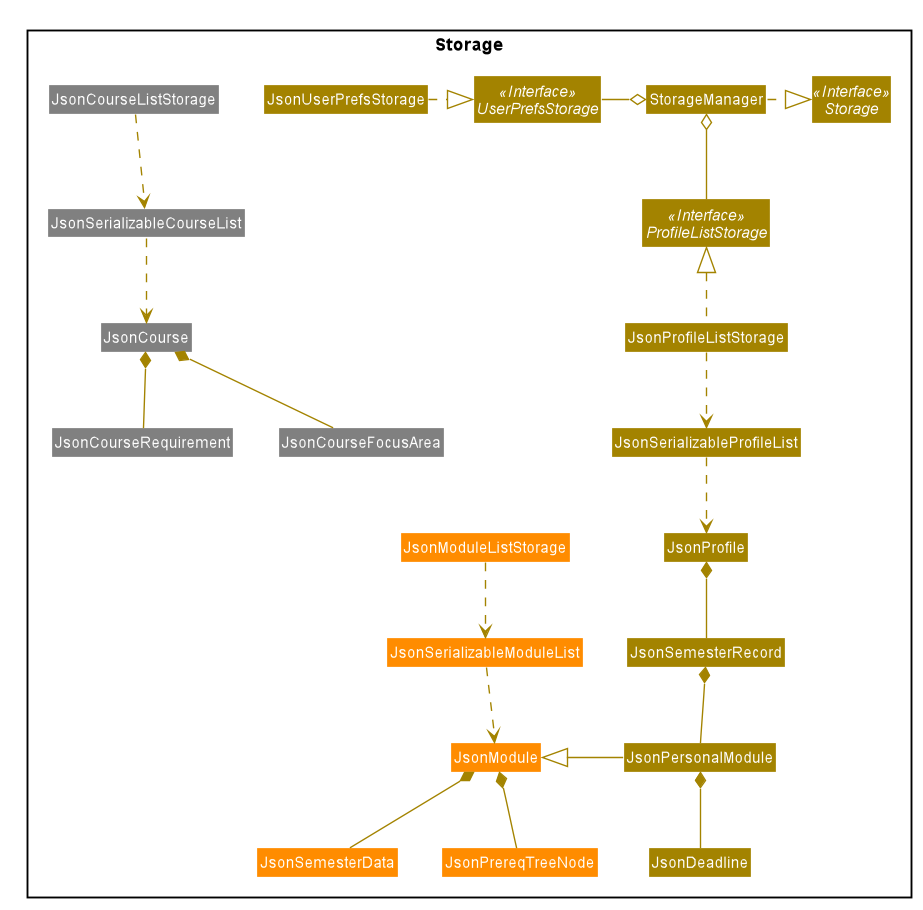
API : Storage.java
The Storage component consists of the following three main parts:
-
Profiles:
JsonProfileListStorage
handles reading and saving of profiles from JSON toProfile
objects and vice versa. TheProfile
objects are stored in aProfileList
. -
Modules:
JsonModuleListStorage
handles only reading of modules from JSON toModule
objects, which are stored in aModuleList
. -
Courses:
JsonCourseListStorage
handles only reading of courses from JSON toCourse
objects, which are stored in aCourseList
.
The Storage
component,
-
can save
UserPref
objects in JSON format and read it back. -
can save the Profile List data in JSON format and read it back as a
ProfileList
object. -
can read Module List data from JSON format to a
ModuleList
object. -
can read Course List data from JSON format to a
CourseList
object.
Delete Profile / Module / Task / Grade Feature
The delete
feature allows the user to delete a Profile
, Module
, Task
or Grade
with the command delete
, appended with the tags.
The tags are:
-
n/name
for deleting aProfile
-
m/moduleCode
for deleting aModule
-
m/moduleCode t/task
for deleting aTask
-
m/moduleCode g/
for deleting aGrade
Multiple modules can be deleted at once, e.g. delete m/CS1231 m/IS1103 m/MA1521 .Multiple tasks can be deleted at once but only from the same module, e.g. delete m/CS1231 t/task t/exam .Multiple grades can be deleted at once, e.g. delete m/CS1231 m/IS1103 m/MA1521 g/ .
|
Current Implementations
DeleteCommand
extends from the Command
class and uses the inheritance to facilitate the implementation. DeleteCommand
is parsed using DeleteCommandParser
to split the user input into relevant fields.
The following sequence diagram shows how the delete
operation works with input: delete m/CS2101 t/work
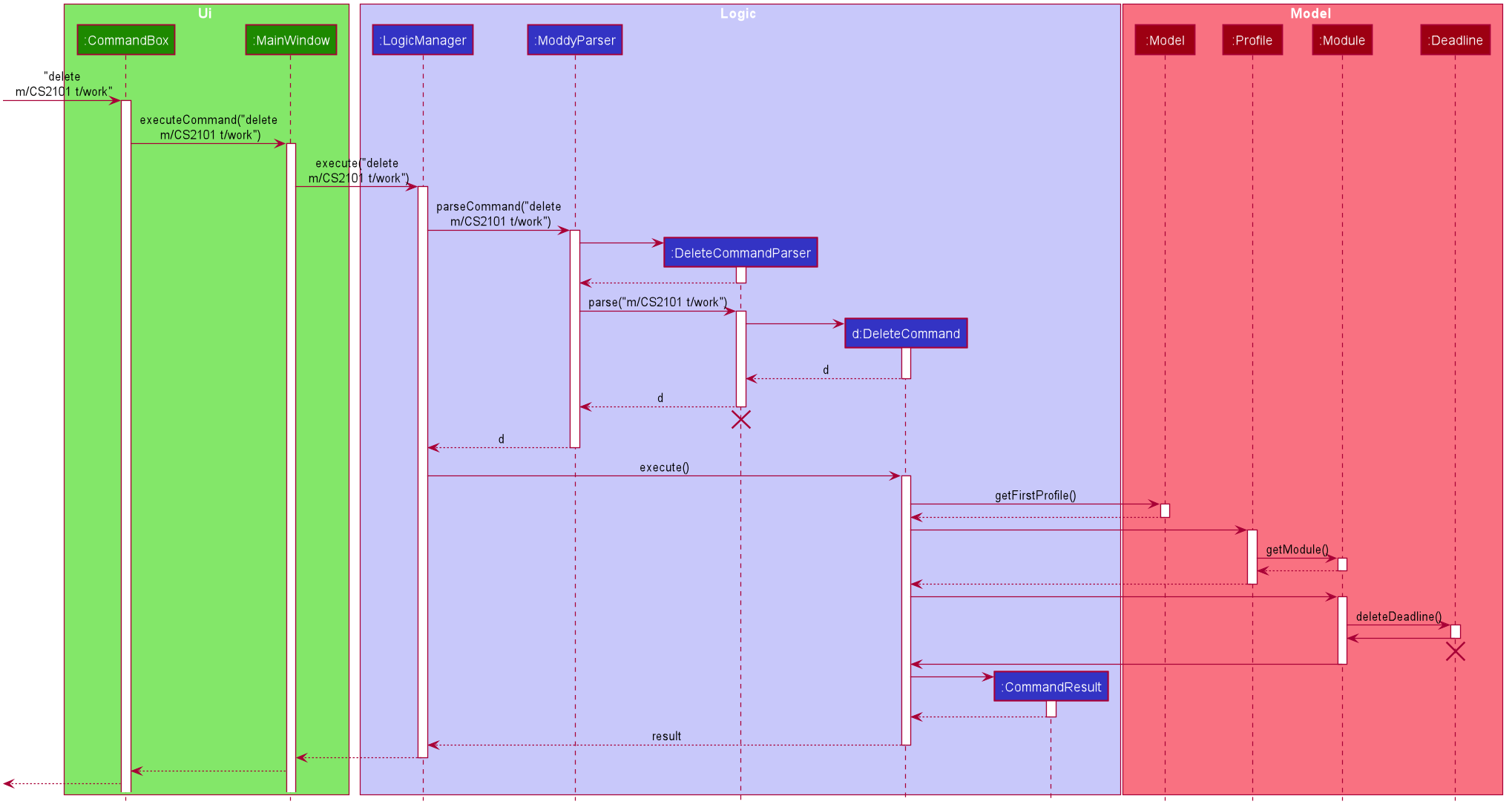
delete m/CS2101 t/work
command.The following activity diagram shows how the show
command decides what to show (profile, module details, semester, course, module or focus area):
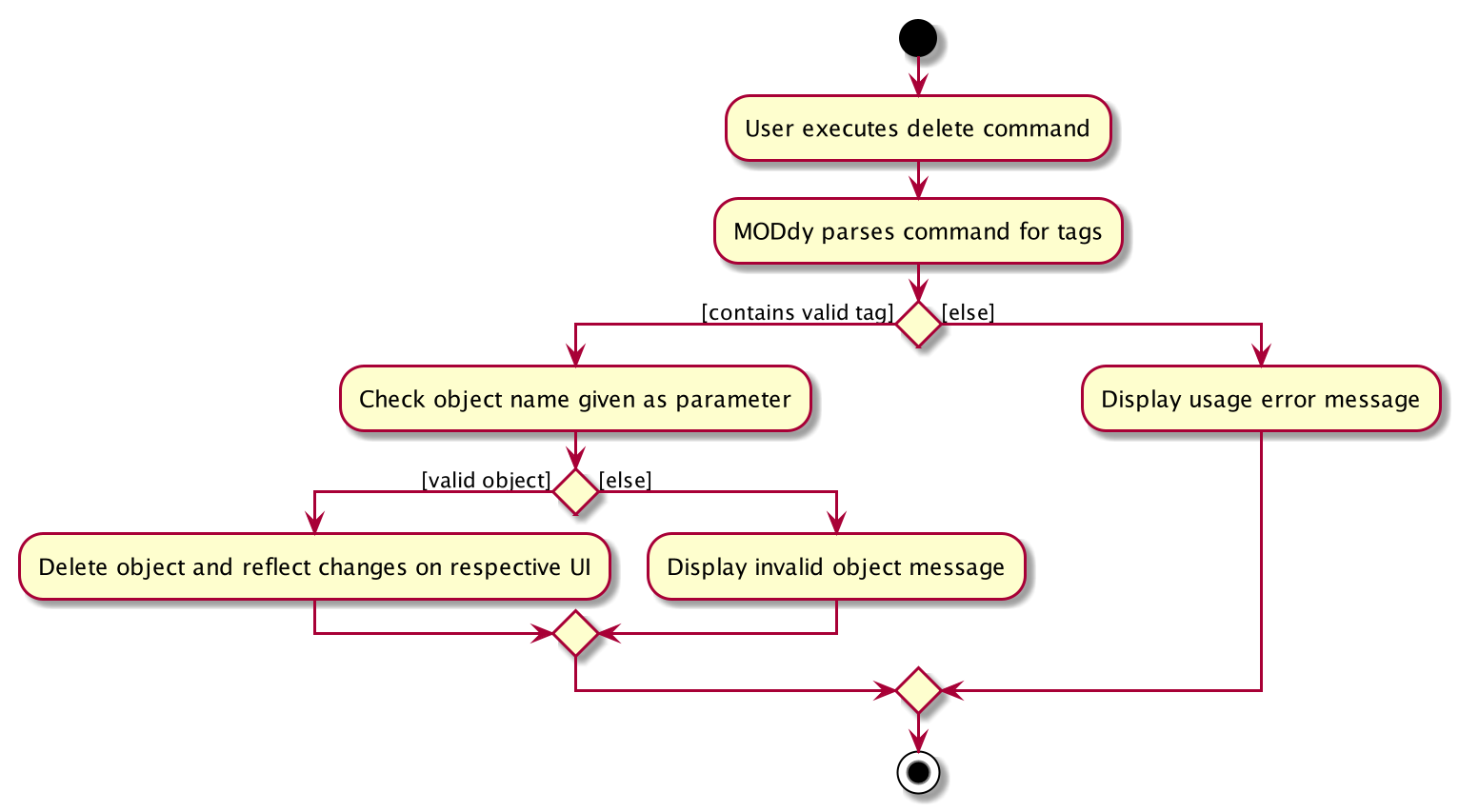
Design Considerations
-
Alternative 1 (current choice): Delete multiple
Module
orTask
objects with oneDeleteCommand
-
Pros: More convenient for users.
-
Cons: Inconsistent with deleting a
Profile
, which can only take in at most oneProfile
.
-
-
Alternative 2: Delete only one
Module
orTask
object with oneDeleteCommand
-
Pros: Easier to implement and consistent with deleting
Profile
. -
Cons: More to type if user intends to delete multiple
Module
objects orTask
objects.
-
Eventually, we decided on alternative 1 to provide more convenience and flexibility to users.
User Stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a … | I want to … | So that I can… |
---|---|---|---|
|
Student |
plan for specialisations |
complete the requirements for my focus area |
|
Student |
see the overview of my degree progression |
|
|
Student |
choose modules to be taken |
plan for future academic semesters |
|
Student |
store my past grades |
calculate my overall CAP |
|
Student |
view prerequisites of every module |
know what modules I should complete early |
|
Student |
view preclusions of every module |
know what modules I cannot take |
|
Student |
maintain a list of unfinished homework and their deadlines |
submit my assignments on time |
|
Student |
edit my list of tasks |
make relevant changes if required |
|
Double degree student |
have a single platform to see both degrees' modules |
track my degree progression |
|
Student |
pool notes for my modules together |
organise my notes according to my modules |
Instructions for Manual Testing
(Content omitted for brevity)